Python: Find the n maximum elements from the provided list
Get N Maximum Elements
Write a Python program to get the n maximum elements from a given list of numbers.
- Use sorted() to sort the list.
- Use slice notation to get the specified number of elements.
- Omit the second argument, n, to get a one-element list.
- If n is greater than or equal to the provided list's length, then return the original list (sorted in descending order).
Sample Solution:
Python Code:
# Define a function 'max_n_nums' that takes a list 'nums' and an optional parameter 'n' (defaulting to 1).
# The function sorts the 'nums' list in descending order and returns the top 'n' maximum values.
def max_n_nums(nums, n=1):
return sorted(nums, reverse=True)[:n]
# Example 1: Create a list 'nums' with elements [1, 2, 3].
nums = [1, 2, 3]
print("Original list elements:")
print(nums)
# Call 'max_n_nums' to find the maximum value in the list.
print("Maximum values of the said list:", max_n_nums(nums))
# Example 2: Create another list 'nums' with elements [1, 2, 3].
nums = [1, 2, 3]
print("\nOriginal list elements:")
print(nums)
# Call 'max_n_nums' with 'n=2' to find the top two maximum values in the list.
print("Two maximum values of the said list:", max_n_nums(nums, 2))
# Example 3: Create a list 'nums' with negative and zero values.
nums = [-2, -3, -1, -2, -4, 0, -5]
print("\nOriginal list elements:")
print(nums)
# Call 'max_n_nums' with 'n=3' to find the top three maximum values in the list.
print("Three maximum values of the said list:", max_n_nums(nums, 3))
# Example 4: Create a list 'nums' with floating-point values.
nums = [2.2, 2, 3.2, 4.5, 4.6, 5.2, 2.9]
print("\nOriginal list elements:")
print(nums)
# Call 'max_n_nums' with 'n=2' to find the top two maximum values in the list.
print("Two maximum values of the said list:", max_n_nums(nums, 2))
Sample Output:
Original list elements: [1, 2, 3] Maximum values of the said list: [3] Original list elements: [1, 2, 3] Two maximum values of the said list: [3, 2] Original list elements: [-2, -3, -1, -2, -4, 0, -5] Threee maximum values of the said list: [0, -1, -2] Original list elements: [2.2, 2, 3.2, 4.5, 4.6, 5.2, 2.9] Two maximum values of the said list: [5.2, 4.6]
Flowchart:
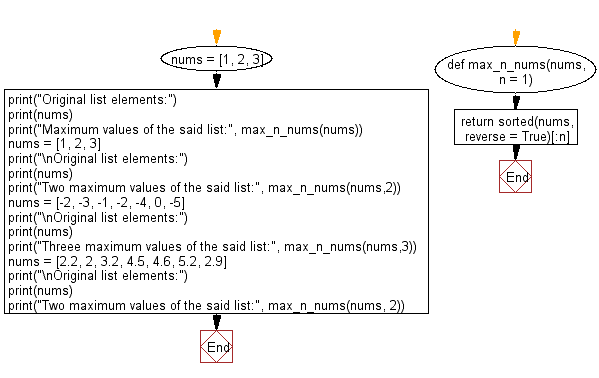
For more Practice: Solve these Related Problems:
- Write a Python program to retrieve the n largest unique elements from a list while preserving their original order.
- Write a Python program to find the n maximum elements from a list and then compute their average.
- Write a Python program to extract the n highest values from a list after filtering out negative numbers.
- Write a Python program to get the n maximum elements from a list of floating-point numbers, rounding them to two decimals before comparison.
Go to:
Previous: Write a Python program to initialize and fills a list with the specified value.
Next: Write a Python program to get the n minimum elements from a given list of numbers.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.