Python: Find the n minimum elements from the provided list
Python List: Exercise - 253 with Solution
Get N Minimum Elements
Write a Python program to get the n minimum elements from a given list of numbers.
- Use sorted() to sort the list.
- Use slice notation to get the specified number of elements.
- Omit the second argument, n, to get a one-element list.
- If n is greater than or equal to the provided list's length, then return the original list (sorted in ascending order).
Sample Solution:
Python Code:
# Define a function 'min_n_nums' that takes a list 'nums' and an optional parameter 'n' (defaulting to 1).
# The function sorts the 'nums' list in ascending order and returns the top 'n' minimum values.
def min_n_nums(nums, n=1):
return sorted(nums, reverse=False)[:n]
# Example 1: Create a list 'nums' with elements [1, 2, 3].
nums = [1, 2, 3]
print("Original list elements:")
print(nums)
# Call 'min_n_nums' to find the minimum value in the list.
print("Minimum values of the said list:", min_n_nums(nums))
# Example 2: Create another list 'nums' with elements [1, 2, 3].
nums = [1, 2, 3]
print("\nOriginal list elements:")
print(nums)
# Call 'min_n_nums' with 'n=2' to find the top two minimum values in the list.
print("Two minimum values of the said list:", min_n_nums(nums, 2))
# Example 3: Create a list 'nums' with negative and zero values.
nums = [-2, -3, -1, -2, -4, 0, -5]
print("\nOriginal list elements:")
print(nums)
# Call 'min_n_nums' with 'n=3' to find the top three minimum values in the list.
print("Three minimum values of the said list:", min_n_nums(nums, 3))
# Example 4: Create a list 'nums' with floating-point values.
print("\nOriginal list elements:")
nums = [2.2, 2, 3.2, 4.5, 4.6, 5.2, 2.9]
print(nums)
# Call 'min_n_nums' with 'n=2' to find the top two minimum values in the list.
print("Two minimum values of the said list:", min_n_nums(nums, 2))
Sample Output:
Original list elements: [1, 2, 3] Minimum values of the said list: [1] Original list elements: [1, 2, 3] Two minimum values of the said list: [1, 2] Original list elements: [-2, -3, -1, -2, -4, 0, -5] Threee minimum values of the said list: [-5, -4, -3] Original list elements: [2.2, 2, 3.2, 4.5, 4.6, 5.2, 2.9] Two minimum values of the said list: [2, 2.2]
Flowchart:
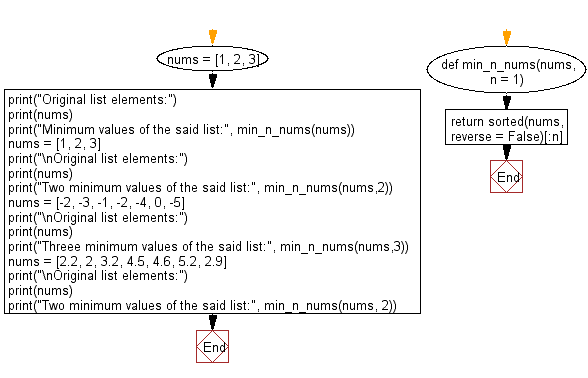
Python Code Editor:
Previous: Write a Python program to get the n maximum elements from a given list of numbers.
Next: Write a Python program to get the weighted average of two or more numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-253.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics