Python: Generate the combinations of n distinct objects taken from a list
Python List: Exercise - 82 with Solution
Write a Python program to generate combinations of n distinct objects taken from the elements of a given list.
Visual Presentation:
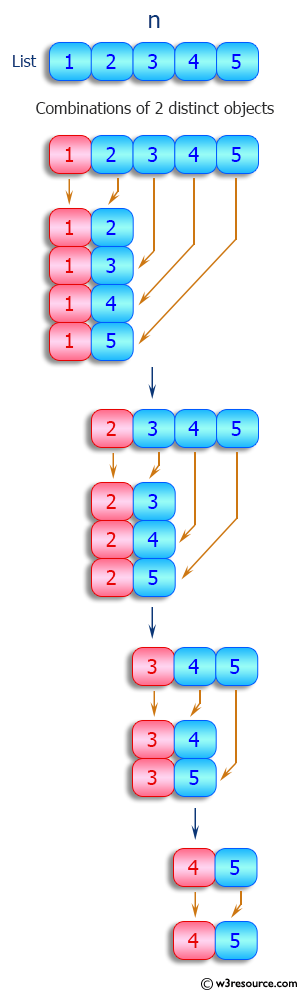
Sample Solution:
Python Code:
# Define a function 'combination' that generates combinations of 'n' distinct objects from 'n_list'
def combination(n, n_list):
# Base case: If 'n' is less than or equal to 0, yield an empty list and return
if n <= 0:
yield []
return
# Iterate through the elements in 'n_list'
for i in range(len(n_list)):
# Take one element 'c_num' at a time starting from index 'i'
c_num = n_list[i:i+1]
# Recursively generate combinations of 'n-1' distinct objects from the remaining elements
for a_num in combination(n-1, n_list[i+1:]):
# Yield the current element 'c_num' combined with the combinations of 'n-1' elements 'a_num'
yield c_num + a_num
# Create a list 'n_list' containing integers
n_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Print a message indicating the original list
print("Original list:")
# Print the original list
print(n_list)
# Assign an integer 'n' with the value 2
n = 2
# Call the 'combination' function with 'n' and 'n_list' and store the generator in the 'result' variable
result = combination(n, n_list)
# Print a message indicating the combinations of 'n' distinct objects
print("\nCombinations of", n, "distinct objects:")
# Iterate through the generator and print each combination
for e in result:
print(e)
Sample Output:
Original list: [1, 2, 3, 4, 5, 6, 7, 8, 9] Combinations of 2 distinct objects: [1, 2] [1, 3] [1, 4] [1, 5] [1, 6] [1, 7] [1, 8] [1, 9] [2, 3] [2, 4] [2, 5] [2, 6] [2, 7] [2, 8] [2, 9] [3, 4] [3, 5] [3, 6] [3, 7] [3, 8] [3, 9] [4, 5] [4, 6] [4, 7] [4, 8] [4, 9] [5, 6] [5, 7] [5, 8] [5, 9] [6, 7] [6, 8] [6, 9] [7, 8] [7, 9] [8, 9]
Flowchart:
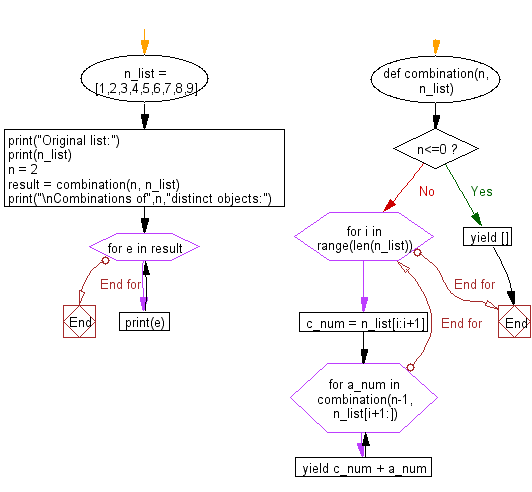
Python Code Editor:
Previous: Write a Python program to extract a given number of randomly selected elements from a given list.
Next: Write a Python program to round every number of a given list of numbers and print the total sum multiplied by the length of the list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics