Python: Find the list with maximum and minimum length
Python List: Exercise - 91 with Solution
Find List with Max and Min Lengths
Write a Python program to find a list with maximum and minimum lengths.
Visual Presentation:
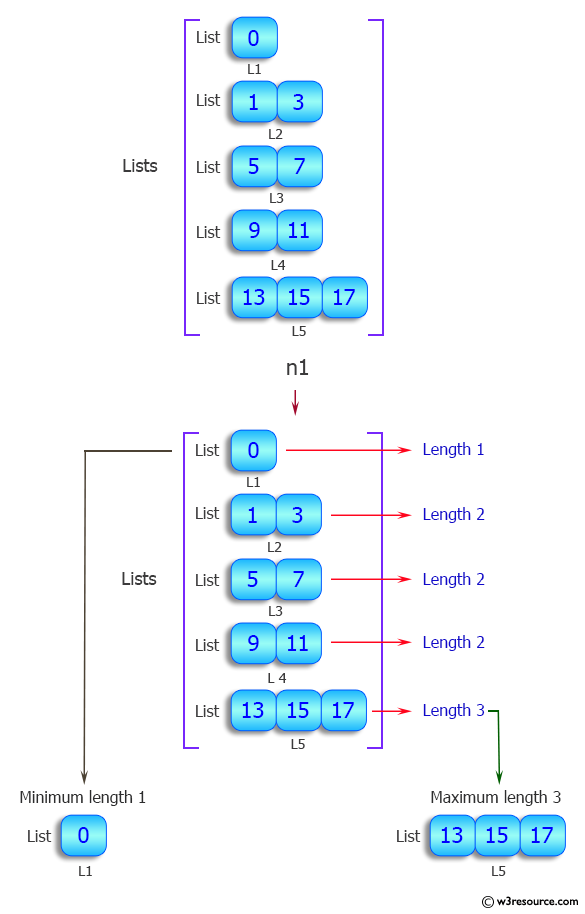
Sample Solution:
Python Code:
# Define a function 'max_length_list' that finds the maximum length and the list with the maximum length in 'input_list'
def max_length_list(input_list):
# Find the maximum length of lists in 'input_list'
max_length = max(len(x) for x in input_list)
# Find the list with the maximum length in 'input_list'
max_list = max(input_list, key=len)
return (max_length, max_list)
# Define a function 'min_length_list' that finds the minimum length and the list with the minimum length in 'input_list'
def min_length_list(input_list):
# Find the minimum length of lists in 'input_list'
min_length = min(len(x) for x in input_list)
# Find the list with the minimum length in 'input_list'
min_list = min(input_list, key=len)
return (min_length, min_list)
# Create a list 'list1' containing sublists of varying lengths
list1 = [[0], [1, 3], [5, 7], [9, 11], [13, 15, 17]]
# Print a message indicating the original list
print("Original list:")
# Print the contents of 'list1'
print(list1)
# Print a message indicating the list with the maximum length of sublists
print("\nList with maximum length of lists:")
# Call the 'max_length_list' function with 'list1' and print the result
print(max_length_list(list1))
# Print a message indicating the list with the minimum length of sublists
print("\nList with minimum length of lists:")
# Call the 'min_length_list' function with 'list1' and print the result
print(min_length_list(list1))
# Create a different list 'list1' containing sublists of varying lengths
list1 = [[0], [1, 3], [5, 7], [9, 11], [3, 5, 7]]
# Print a message indicating the original list
print("Original list:")
# Print the contents of the new 'list1'
print(list1)
# Print a message indicating the list with the maximum length of sublists
print("\nList with maximum length of lists:")
# Call the 'max_length_list' function with the new 'list1' and print the result
print(max_length_list(list1))
# Print a message indicating the list with the minimum length of sublists
print("\nList with minimum length of lists:")
# Call the 'min_length_list' function with the new 'list1' and print the result
print(min_length_list(list1))
# Create another list 'list1' containing sublists of varying lengths
list1 = [[12], [1, 3], [1, 34, 5, 7], [9, 11], [3, 5, 7]]
# Print a message indicating the original list
print("Original list:")
# Print the contents of the new 'list1'
print(list1)
# Print a message indicating the list with the maximum length of sublists
print("\nList with maximum length of lists:")
# Call the 'max_length_list' function with the new 'list1' and print the result
print(max_length_list(list1))
# Print a message indicating the list with the minimum length of sublists
print("\nList with minimum length of lists:")
# Call the 'min_length_list' function with the new 'list1' and print the result
print(min_length_list(list1))
Sample Output:
Original list: [[0], [1, 3], [5, 7], [9, 11], [13, 15, 17]] List with maximum length of lists: (3, [13, 15, 17]) List with minimum length of lists: (1, [0]) Original list: [[0], [1, 3], [5, 7], [9, 11], [3, 5, 7]] List with maximum length of lists: (3, [3, 5, 7]) List with minimum length of lists: (1, [0]) Original list: [[12], [1, 3], [1, 34, 5, 7], [9, 11], [3, 5, 7]] List with maximum length of lists: (4, [1, 34, 5, 7]) List with minimum length of lists: (1, [12])
Flowchart:
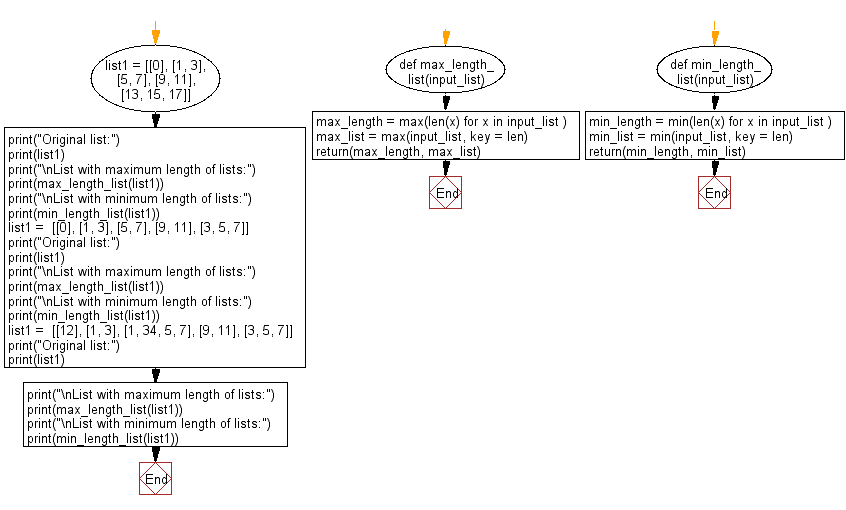
Python Code Editor:
Previous: Write a Python program to count number of lists in a given list of lists.
Next: Write a Python program to check if a nested list is a subset of another nested list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-91.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics