Python Math: Find the smallest multiple of the first n numbers
10. Smallest Multiple and Factors
Write a Python program to find the smallest multiple of the first n numbers. Also, display the factors.
Sample Solution:
Python Code:
def smallest_multiple(n):
if (n<=2):
return n
i = n * 2
factors = [number for number in range(n, 1, -1) if number * 2 > n]
print(factors)
while True:
for a in factors:
if i % a != 0:
i += n
break
if (a == factors[-1] and i % a == 0):
return i
print(smallest_multiple(13))
print(smallest_multiple(11))
print(smallest_multiple(2))
print(smallest_multiple(1))
Sample Output:
[13, 12, 11, 10, 9, 8, 7] 360360 [11, 10, 9, 8, 7, 6] 27720 2 1
Flowchart:
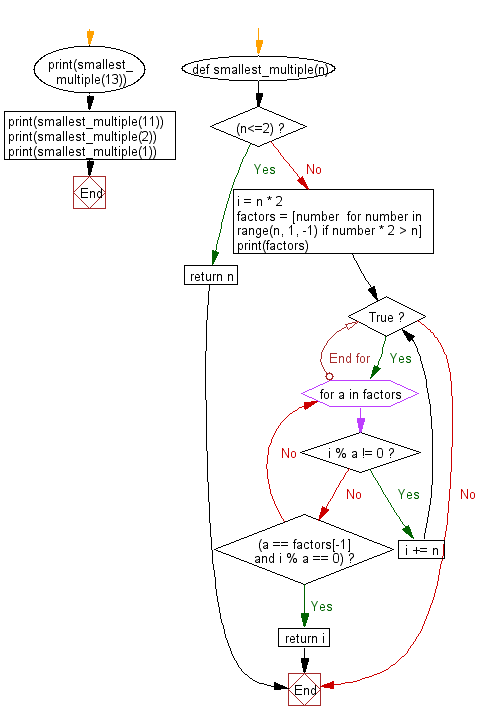
For more Practice: Solve these Related Problems:
- Write a Python program that finds the smallest common multiple of the first n natural numbers and prints the factors that contribute to it.
- Write a Python function to calculate the least common multiple (LCM) of numbers from 1 to n and display its prime factorization.
- Write a Python script to compute the smallest multiple for a given n, then list all factors of that multiple in descending order.
- Write a Python program to determine the smallest number divisible by all numbers from 1 to n and verify its divisibility using a loop.
Go to:
Previous: Write a Python program to calculate the discriminant value.
Next: Write a Python program to calculate the difference between the squared sum of first n natural numbers and the sum of squared first n natural numbers.(default value of number=2).
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.