Performing element-wise addition in Pandas DataFrame with NumPy array
Python Pandas Numpy: Exercise-9 with Solution
Perform element-wise addition of a NumPy array and a Pandas DataFrame column.
Sample Solution:
Python Code:
import pandas as pd
import numpy as np
# Create a sample DataFrame
data = {'Name': ['Teodosija', 'Sutton', 'Taneli', 'David', 'Ross'],
'Age': [25, 30, 22, 35, 28],
'Salary': [50000, 60000, 45000, 70000, 55000]}
df = pd.DataFrame(data)
# Create a NumPy array
numpy_array = np.array([1000, 2000, 3000, 4000, 5000])
# Perform element-wise addition using numpy.add()
df['Updated_Salary'] = np.add(df['Salary'], numpy_array)
# Display the updated DataFrame
print(df)
Output:
Name Age Salary Updated_Salary 0 Teodosija 25 50000 51000 1 Sutton 30 60000 62000 2 Taneli 22 45000 48000 3 David 35 70000 74000 4 Ross 28 55000 60000
Explanation:
In the exerciser above -
- First we create a sample DataFrame (df) with columns 'Name', 'Age', and 'Salary'.
- Next we create a NumPy array numpy_array with values to add element-wise to the 'Salary' column.
- The numpy.add(df['Salary'], numpy_array) function performs element-wise addition, and the result is assigned to a new column 'Updated_Salary'.
- The updated DataFrame is then printed to the console.
Flowchart:
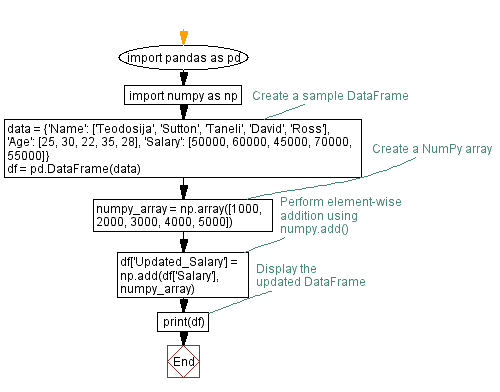
Python Code Editor:
Previous: Filtering DataFrame rows by column values in Pandas using NumPy array.
Next: Applying NumPy function to DataFrame column in Python.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics