Applying NumPy function to DataFrame column in Python
Python Pandas Numpy: Exercise-10 with Solution
Apply a NumPy function to a Pandas DataFrame column.
Sample Solution:
Python Code:
import pandas as pd
import numpy as np
# Create a sample DataFrame
data = {'Name': ['Teodosija', 'Sutton', 'Taneli', 'David', 'Ross'],
'Age': [25, 30, 22, 35, 28],
'Salary': [50000, 60000, 45000, 70000, 55000]}
df = pd.DataFrame(data)
# Define a NumPy function (e.g., np.sqrt) to apply to the 'Salary' column
df['Sqrt_Salary'] = np.sqrt(df['Salary'])
# Display the DataFrame with the new column
print(df)
Output:
Name Age Salary Sqrt_Salary 0 Teodosija 25 50000 223.606798 1 Sutton 30 60000 244.948974 2 Taneli 22 45000 212.132034 3 David 35 70000 264.575131 4 Ross 28 55000 234.520788
Explanation:
In the exerciser above -
- First we create a sample DataFrame (df) with columns 'Name', 'Age', and 'Salary'.
- Next we use the NumPy function np.sqrt() to calculate the square root of each element in the 'Salary' column.
- The result is assigned to a new column 'Sqrt_Salary'.
- The updated DataFrame is then printed to the console.
Flowchart:
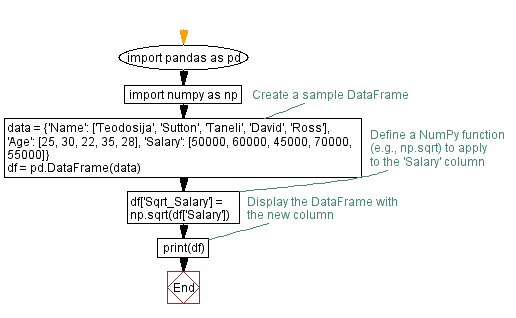
Python Code Editor:
Previous: Performing element-wise addition in Pandas DataFrame with NumPy array.
Next: Calculating correlation matrix for DataFrame in Python.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics