Capture and display mouse events in PyQt5 with Python
Python PyQt Event Handling: Exercise-2 with Solution
Write a Python program that captures and displays mouse events like clicks and movements using PyQt5.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QLabel Class: The QLabel widget provides a text or image display.
QWidget Class: The QWidget class is the base class of all user interface objects.
Qt module: PyQt5 is a set of Python bindings for the Qt application framework. It allows us to use Qt, a popular C++ framework, to create graphical user interfaces (GUIs) in Python.
QEvent Class: The QEvent class is the base class of all event classes. Event objects contain event parameters.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QWidget
from PyQt5.QtCore import Qt, QEvent
class MouseEventApp(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Mouse Event Example")
self.setGeometry(100, 100, 400, 200)
self.central_widget = QWidget(self)
self.setCentralWidget(self.central_widget)
self.mouse_label = QLabel("Mouse Events:", self.central_widget)
self.mouse_label.setGeometry(10, 10, 200, 30)
self.mouse_tracker_label = QLabel("", self.central_widget)
self.mouse_tracker_label.setGeometry(10, 40, 300, 30)
# Install an event filter to capture mouse events
self.central_widget.installEventFilter(self)
def eventFilter(self, obj, event):
if event.type() == QEvent.MouseMove:
x = event.x()
y = event.y()
self.mouse_tracker_label.setText(f"Mouse moved to ({x}, {y})")
elif event.type() == QEvent.MouseButtonPress:
button = ""
if event.button() == Qt.LeftButton:
button = "Left"
elif event.button() == Qt.RightButton:
button = "Right"
elif event.button() == Qt.MiddleButton:
button = "Middle"
self.mouse_tracker_label.setText(f"{button} button clicked")
return super().eventFilter(obj, event)
def main():
app = QApplication(sys.argv)
window = MouseEventApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary PyQt5 modules.
- Create a custom class MouseEventApp that inherits from QMainWindow.
- Inside the MouseEventApp class constructor (__init__), we set up the main window, labels to display mouse events, and install an event filter on the central widget.
- The eventFilter() function captures mouse events, specifically mouse movements and button clicks. When a mouse event occurs, the labels are updated accordingly.
- We create an instance of the MouseEventApp class, show the main window, and start the PyQt5 application event loop.
Output:
Flowchart:
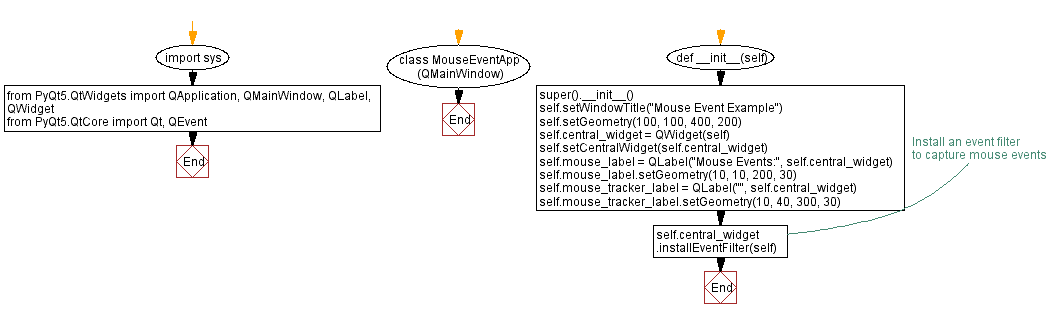
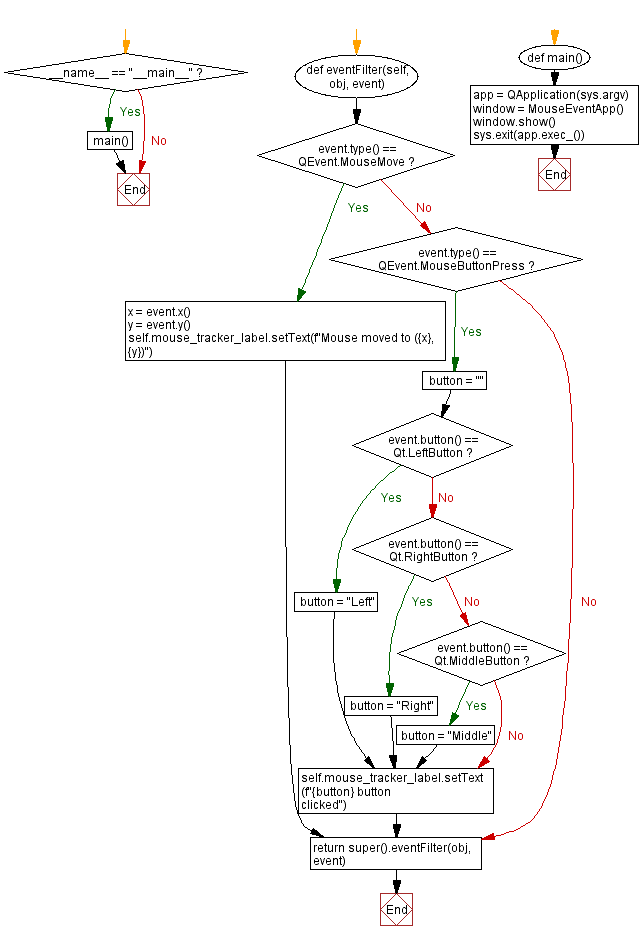
Python Code Editor:
Previous: Event-Driven programming in PyQt5 with Python.
Next: Python PyQt keyboard event handling example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/pyqt/python-pyqt-event-handling-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics