Event-Driven programming in PyQt5 with Python
Write a Python exercise that explain the concept of event-driven programming in PyQt5.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QPushButton Class: The QPushButton widget provides a command button.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton
# Create a custom function to handle a button click event
def button_clicked():
print("Button was clicked!")
# Create the PyQt5 application
app = QApplication(sys.argv)
# Create a main window
window = QMainWindow()
window.setWindowTitle("Event-Driven Programming Example")
window.setGeometry(100, 100, 400, 200)
# Create a QPushButton (a clickable button)
button = QPushButton("Click Me!", window)
button.setGeometry(150, 80, 100, 30)
# Connect the button's clicked signal to the custom function
button.clicked.connect(button_clicked)
# Show the main window
window.show()
# Start the event loop to listen for events
sys.exit(app.exec_())
Explanation:
In the exercise above -
- Import the necessary PyQt5 modules.
- Create a custom function button_clicked that will handle the button click event.
- Create a PyQt5 application using QApplication.
- Next, we create a main window (QMainWindow) with a title and dimensions.
- Add a "QPushButton" widget labeled "Click Me!" to the main window and position it.
- When the button is clicked, the button_clicked function will be executed.
- Display the main window using window.show().
- Finally, we start the event loop with app.exec_().
Output:
Button was clicked!![]()
Flowchart:
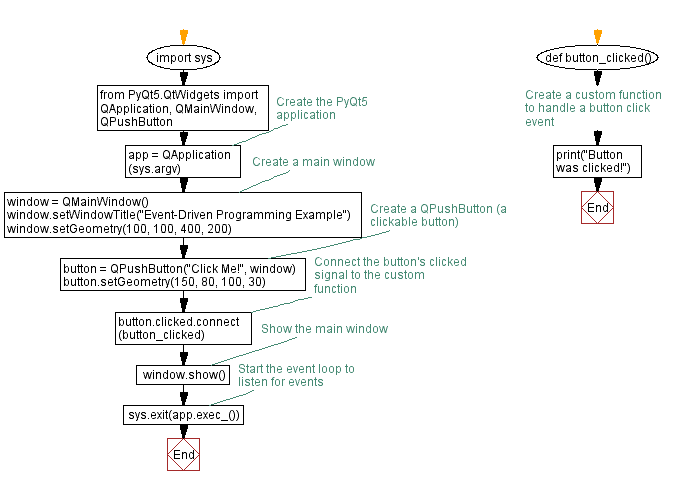
Python Code Editor:
Previous: Python PyQt Event Handling Home.
Next: Capture and display mouse events in PyQt5 with Python.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.