Python PyQt custom button example
Write a Python program to create a window with a custom button using PyQt. When you click the custom button, a message will be printed to the console, demonstrating the changed behavior.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QPushButton Class: The QPushButton widget provides a command button.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton
class CustomButton(QPushButton):
def __init__(self, text, parent=None):
super().__init__(text, parent)
def mousePressEvent(self, event):
# Customize the behavior when the button is clicked
print("'Click Me' button was clicked!")
class CustomButtonApp(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Custom Button Example")
self.setGeometry(100, 100, 400, 200)
custom_button = CustomButton("Click Me!", self)
custom_button.setGeometry(150, 80, 100, 30)
def main():
app = QApplication(sys.argv)
window = CustomButtonApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary PyQt5 modules.
- Create a custom button class called "CustomButton" by subclassing the "QPushButton" widget.
- Inside the CustomButton class, we override the "mousePressEvent()" method. This method is called when the button is clicked. In our custom implementation, it simply prints a message to the console.
- Create an instance of the "CustomButton" class in the "CustomButtonApp" class, which represents our main application window. This custom button behaves differently from a standard button because of its overridden "mousePressEvent" method.
Output:
'Click Me' button was clicked!![]()
Flowchart:
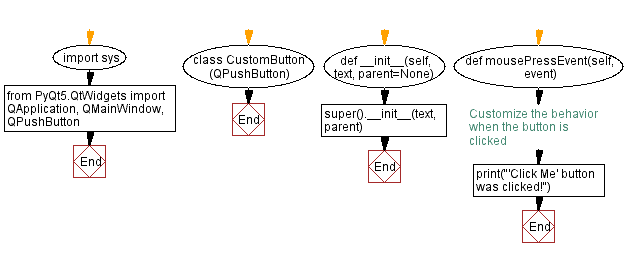
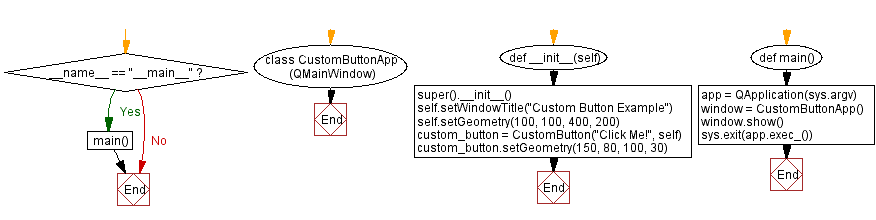
Go to:
Previous: Python PyQt keyboard event handling example.
Next: Python PyQt custom widget with keyboard shortcuts.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.