Creating a custom combobox in Python with Tkinter
Write a Python program that creates a custom combobox widget with a unique dropdown design and custom dropdown items using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
class CustomCombobox:
def __init__(self, root):
self.root = root
self.root.title("Custom Combobox")
# Create a Combobox
self.combobox = ttk.Combobox(root)
# Set custom dropdown items
self.custom_items = ["Python", "Java", "C++", "Other Language"]
self.combobox['values'] = self.custom_items
# Bind a function to execute when an item is selected
self.combobox.bind("<<ComboboxSelected>>", self.on_combobox_select)
# Pack the Combobox
self.combobox.pack(pady=20)
def on_combobox_select(self, event):
selected_item = self.combobox.get()
print(f"Selected item: {selected_item}")
if __name__ == "__main__":
root = tk.Tk()
app = CustomCombobox(root)
root.mainloop()
Explanation:
In the exercise above -
- Create a "ttk.Combobox" widget and set its values to a list of custom items.
- Bind the <<ComboboxSelected>> event to the on_combobox_select function, which is executed when an item is selected from the dropdown.
- When an item is selected, the selected item is printed to the console.
Output:
Selected item: Python Selected item: Java Selected item: C++ Selected item: Other Language![]()
Flowchart:
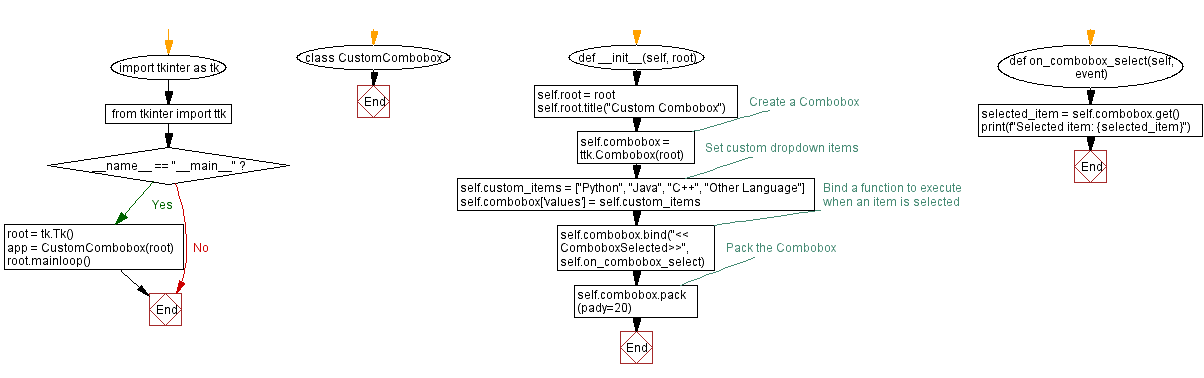

Go to:
Previous: Creating a custom Tabbed interface in Python with Tkinter.
Next: Creating themed labels in Python with Tkinter.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.