Python Tkinter text editor: Save text to file
Python Tkinter Dialogs and File Handling: Exercise-10 with Solution
Write a Python program using Tkinter that allows the user to create a text file and write content to it using a Text widget.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import filedialog
def save_to_file():
file_path = filedialog.asksaveasfilename(defaultextension=".txt", filetypes=[("Text files", "*.txt"), ("All files", "*.*")])
if file_path:
try:
with open(file_path, 'w') as file:
text_content = text_widget.get("1.0", "end-1c")
file.write(text_content)
status_label.config(text=f"File saved: {file_path}")
except Exception as e:
status_label.config(text=f"Error saving file: {str(e)}")
root = tk.Tk()
root.title("Text Editor")
text_widget = tk.Text(root, wrap=tk.WORD)
text_widget.pack(padx=20, pady=20, fill="both", expand=True)
save_button = tk.Button(root, text="Save to File", command=save_to_file)
save_button.pack(pady=10)
status_label = tk.Label(root, text="", padx=20, pady=10)
status_label.pack()
root.mainloop()
Explanation:
In the exercise above -
- Import "tkinter" as "tk" and "filedialog" for creating the GUI components and opening the file save dialog.
- Define the "save_to_file()" function, which opens the file save dialog using "filedialog.asksaveasfilename()" function. This dialog allows the user to specify a file path for saving text. If a file path is selected, it reads the Text widget content using "text_widget.get()" function, and then writes it to the selected file. The status label is updated to show the saved file path or an error message if saving fails.
- Create the main Tkinter window, set its title, and create a Text widget for editing text.
- Create a "Save to File" button that calls the "save_to_file()" function when clicked.
- Create a status label to show messages about file saving or errors.
- The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
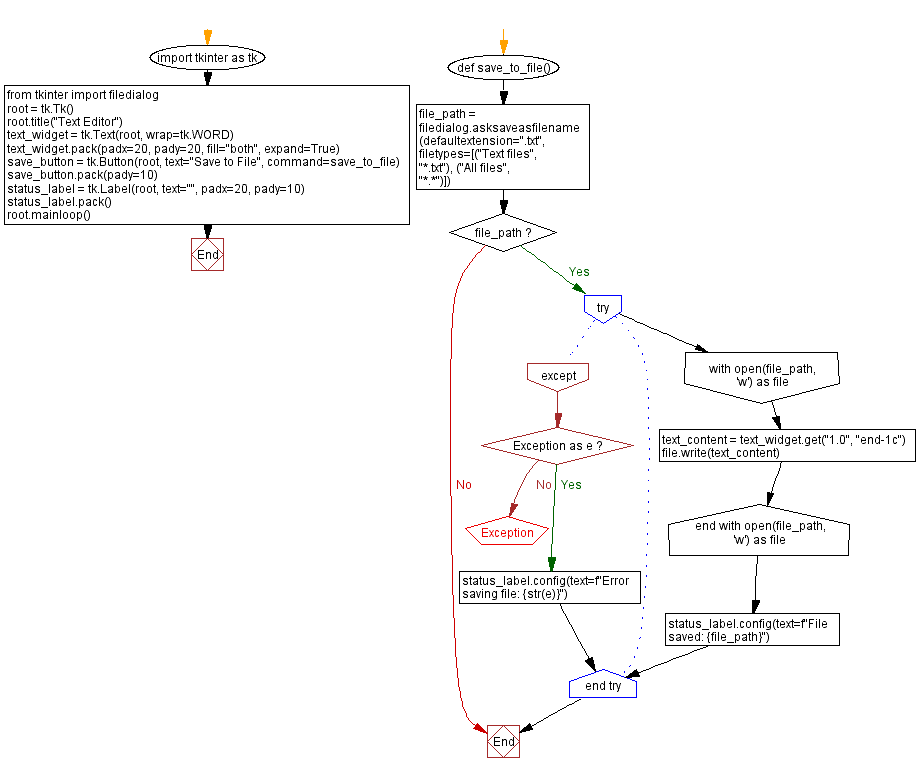
Python Code Editor:
Previous: Python CSV file viewer with Tkinter.
Next: Python Tkinter text editor with file dialogs.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics