Python CSV file viewer with Tkinter
Write a Python program to create an application that reads a CSV file and displays its data in a Tkinter Treeview widget.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
from tkinter import filedialog
import csv
def open_csv_file():
file_path = filedialog.askopenfilename(title="Open CSV File", filetypes=[("CSV files", "*.csv")])
if file_path:
display_csv_data(file_path)
def display_csv_data(file_path):
try:
with open(file_path, 'r', newline='') as file:
csv_reader = csv.reader(file)
header = next(csv_reader) # Read the header row
tree.delete(*tree.get_children()) # Clear the current data
tree["columns"] = header
for col in header:
tree.heading(col, text=col)
tree.column(col, width=100)
for row in csv_reader:
tree.insert("", "end", values=row)
status_label.config(text=f"CSV file loaded: {file_path}")
except Exception as e:
status_label.config(text=f"Error: {str(e)}")
root = tk.Tk()
root.title("CSV File Viewer")
open_button = tk.Button(root, text="Open CSV File", command=open_csv_file)
open_button.pack(padx=20, pady=10)
tree = ttk.Treeview(root, show="headings")
tree.pack(padx=20, pady=20, fill="both", expand=True)
status_label = tk.Label(root, text="", padx=20, pady=10)
status_label.pack()
root.mainloop()
Explanation:
In the exercise above -
- Import "tkinter" as "tk", "ttk" and "filedialog" for creating the GUI components and opening the folder dialog. We also import "csv" for working with CSV files.
- Define the "open_csv_file()" function, which opens the file dialog using "filedialog.askopenfilename()". This dialog allows the user to select a CSV file. If a file is selected, it calls the display_csv_data() function.
- Define the "display_csv_data()" function, which reads the CSV file using the csv.reader and displays its data in a Tkinter Treeview widget. It first clears the current data in the "Treeview" widget, then reads the header row to configure the columns and column headings, and finally inserts the data rows.
- Create the main Tkinter window, set its title, and create an "Open CSV File" button that calls the open_csv_file() function when clicked.
- Create a Treeview widget (tree) to display "CSV" data with column headers.
- Create a status label to show messages about "CSV" file loading or errors.
- The main event loop, "root.mainloop()", starts the Tkinter application.
Sample Output:
Flowchart:
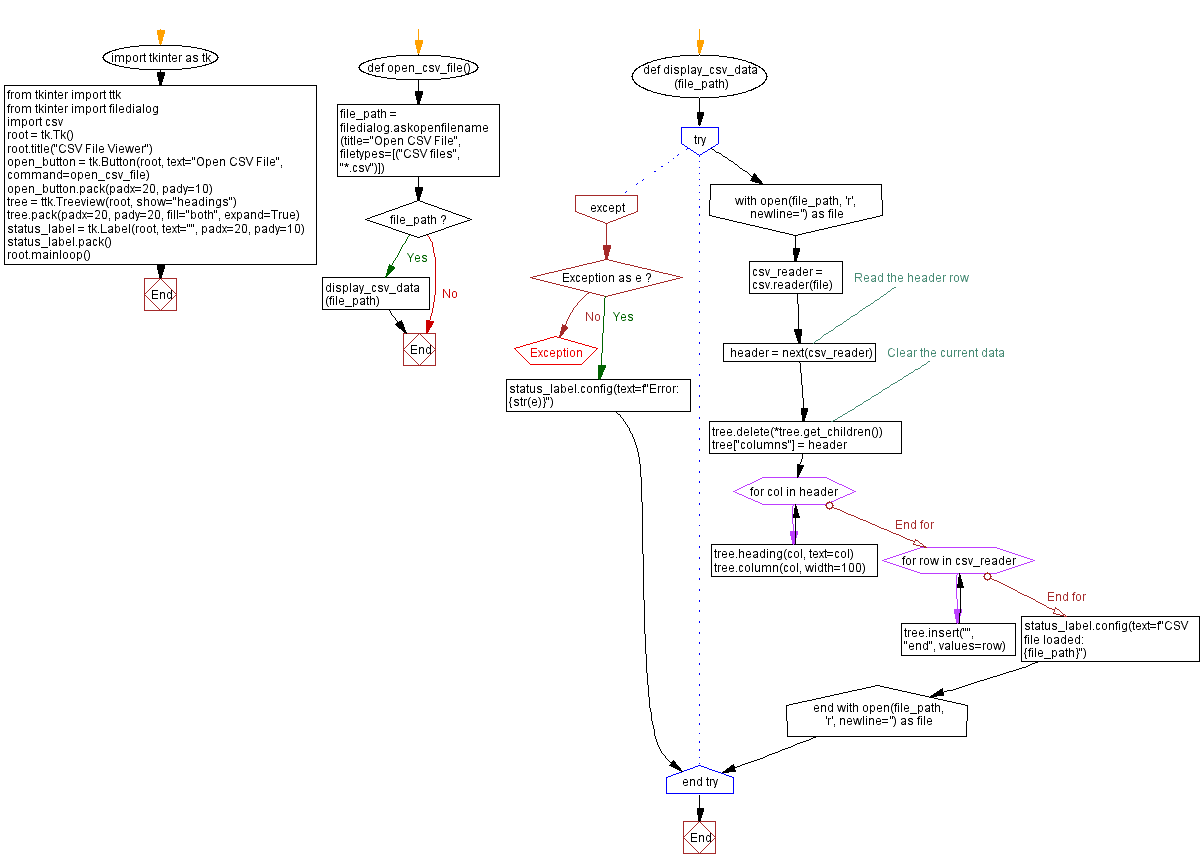
Go to:
Previous: Open and display images.
Next: Save text to file.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.