Python Tkinter: Creating a custom input dialog
Write a Python program to implement a custom input dialog that prompts the user to input their name and displays it in a label using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import simpledialog
def show_name_dialog():
name = simpledialog.askstring("Input", "Input your name:")
if name:
name_label.config(text=f"Hello, {name}!")
parent = tk.Tk()
parent.title("Input Dialog Example")
get_name_button = tk.Button(parent, text="Get Name", command=show_name_dialog)
get_name_button.pack(padx=20, pady=20)
name_label = tk.Label(parent, text="", padx=20, pady=10)
name_label.pack()
parent.mainloop()
Explanation:
In the exercise above -
- Import "tkinter" as "tk" and "simpledialog" for creating the GUI components and the custom input dialog.
- Define the "show_name_dialog()" function, which uses simpledialog.askstring() to display a custom input dialog titled "Input" with a prompt to "Input your name." The user's input (name) is stored in the name variable. If a name is provided, it updates a label with a greeting message.
- Create the main Tkinter window, set its title, and create a button labeled "Get Name" that calls the "show_name_dialog()" function when clicked.
- Create a label to display the greeting message with some padding.
- The main event loop, parent.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
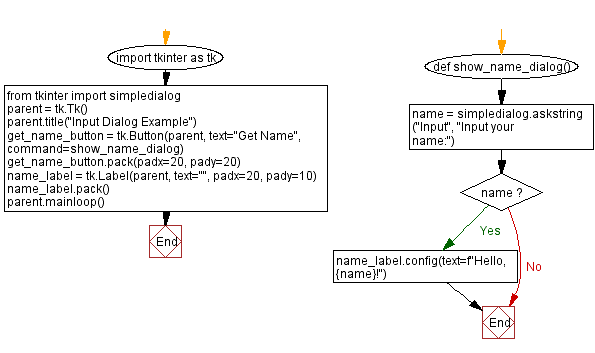
Go to:
Previous: Building a color picker dialog.
Next: List folder contents.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.