Python file explorer with Tkinter: List folder contents
Write a Python program that creates a file explorer application that displays the list of files and directories in a selected folder using Tkinter.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import filedialog
import os
def open_folder_dialog():
folder_path = filedialog.askdirectory(title="Select a Folder")
if folder_path:
display_folder_contents(folder_path)
def display_folder_contents(folder_path):
try:
folder_contents = os.listdir(folder_path)
listbox.delete(0, tk.END) # Clear the current list
for item in folder_contents:
listbox.insert(tk.END, item)
except Exception as e:
listbox.delete(0, tk.END) # Clear the current list
listbox.insert(tk.END, f"Error: {str(e)}")
parent = tk.Tk()
parent.title("File Explorer")
open_button = tk.Button(parent, text="Open Folder", command=open_folder_dialog)
open_button.pack(padx=20, pady=20)
listbox = tk.Listbox(parent, height=15, width=40)
listbox.pack(padx=20, pady=20)
parent.mainloop()
Explanation:
In the exercise above -
- Import "tkinter" as "tk" and "filedialog" for creating the GUI components and opening the folder dialog.
- Import the "os" module to interact with the file system.
- Define the "open_folder_dialog()" function, which opens the folder dialog using "filedialog.askdirectory()" function. This dialog allows the user to select a folder. If a folder is selected, it calls the "display_folder_contents()" function.
- Define the "display_folder_contents()" function, which lists the contents of the selected folder using os.listdir(). It clears the current list in the Listbox and inserts the folder contents into it. It also handles any exceptions that may occur.
- Create the main Tkinter window, set its title, and create the "Open Folder" button, which calls the open_folder_dialog() function when clicked.
- Create a Listbox widget to display the list of files and directories.
- The main event loop, parent.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
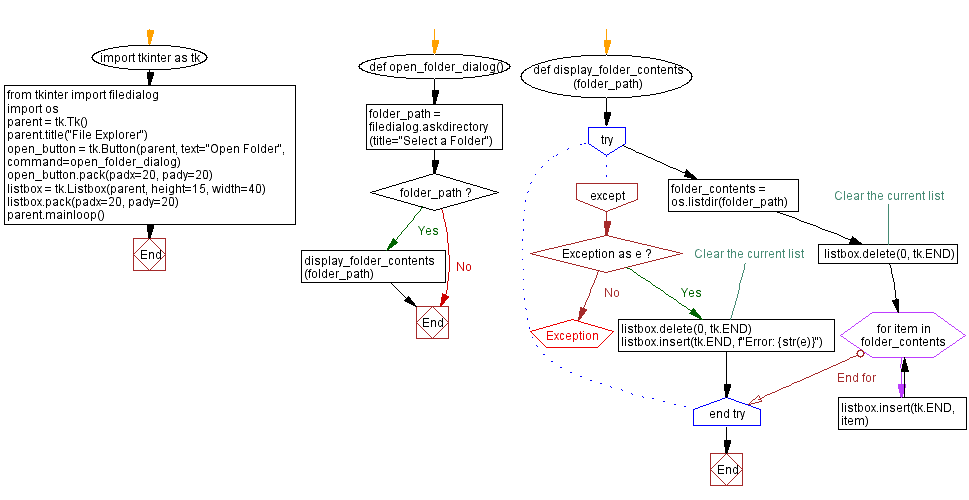
Go to:
Previous: Creating a custom input dialog.
Next: Create and save text files.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.