Python Tkinter text file writer: Create and save text files
Write a Python program using Tkinter that allows the user to create a text file and write content to it using a Text widget.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import filedialog
def save_as_text_file():
file_path = filedialog.asksaveasfilename(defaultextension=".txt", filetypes=[("Text files", "*.txt"), ("All files", "*.*")])
if file_path:
try:
with open(file_path, 'w') as file:
file_content = text_widget.get('1.0', tk.END)
file.write(file_content)
status_label.config(text=f"File saved as: {file_path}")
except Exception as e:
status_label.config(text=f"Error: {str(e)}")
root = tk.Tk()
root.title("Text file writer")
text_widget = tk.Text(root, wrap=tk.WORD, height=15, width=35)
text_widget.pack(padx=20, pady=20)
save_button = tk.Button(root, text="Save As", command=save_as_text_file)
save_button.pack(padx=20, pady=10)
status_label = tk.Label(root, text="", padx=20, pady=10)
status_label.pack()
root.mainloop()
Explanation:
In the exercise above -
- Import "tkinter" as "tk" and "filedialog" for creating the GUI components and opening the folder dialog.
- Define the "save_as_text_file()" function, which opens the file dialog using "filedialog.asksaveasfilename()". This dialog allows the user to specify a file name and location for saving the text file. If a file path is provided, it attempts to save the Text widget content to that file and updates a status label.
- Create the main Tkinter window, set its title, and create a Text widget for entering text content.
- Create a "Save As" button that calls the "save_as_text_file()" function when clicked.
- Create a status label to display messages about file saving or errors.
- The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
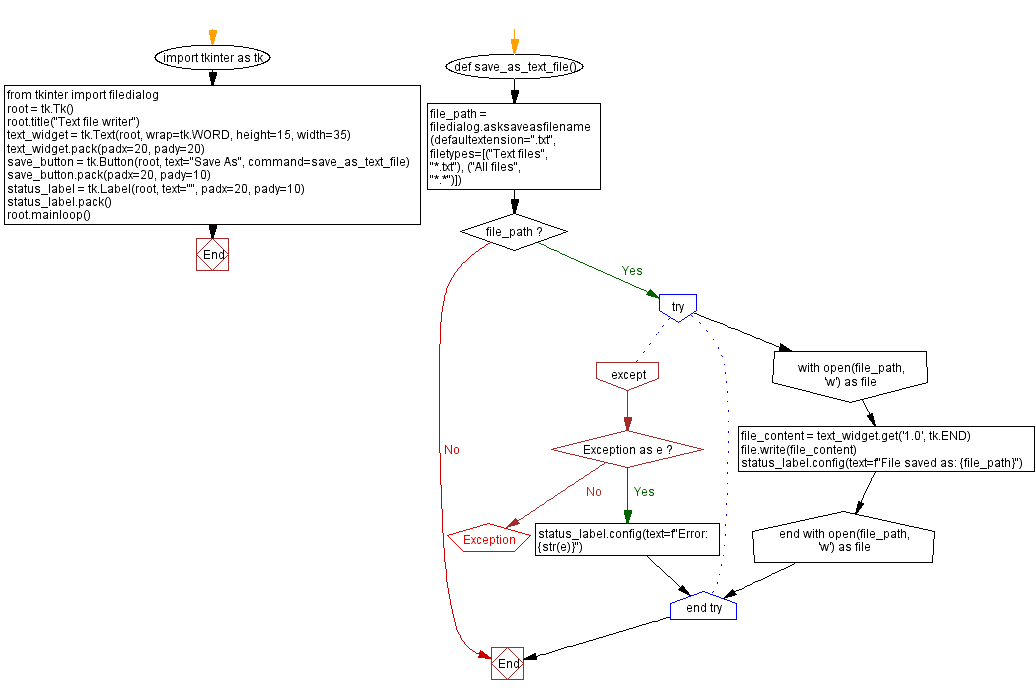
Go to:
Previous: List folder contents.
Next: Open and display images.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.