Python Event Hooks for Request and Response Customization
Write a Python program that uses event hooks for both requests and responses to customize behavior at various stages of the request/response lifecycle.
Sample Solution:
Python Code :
import urllib3
# Event hook for modifying request headers before sending
def modify_request_headers(request_headers):
print("Modifying request headers...")
request_headers['User-Agent'] = 'Custom User-Agent'
return request_headers
# Event hook for handling response data after receiving
def handle_response(response):
print("Handling response...")
print(f"Response status code: {response.status}")
print("Response data:")
print(response.data.decode('utf-8'))
# Create a PoolManager with event hooks
http = urllib3.PoolManager()
http.headers_callback = modify_request_headers
http.response_callback = handle_response
# Define the URL for the request
url = 'https://www.example.com'
try:
# Make a GET request
response = http.request('GET', url)
# Check if the request was successful (status code 200)
if response.status == 200:
print("Request Successful!")
else:
print(f"Error: Unable to fetch data. Status Code: {response.status}")
except Exception as e:
print(f"Error: {e}")
Sample Output:
Request Successful!
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- We define two event hooks: "modify_request_headers" for modifying request headers before sending and "handle_response" for handling response data after receiving.
- We create a 'PoolManager' object and assign event hooks to "http.headers_callback" and "http.response_callback".
- When a request is made, the "modify_request_headers()" function is called to modify the request headers before sending.
- After receiving the response, the "handle_response()" function is called to handle the response data.
- Finally, we make a GET request to the specified URL, and the defined event hooks are invoked during the request/response lifecycle.
Flowchart:
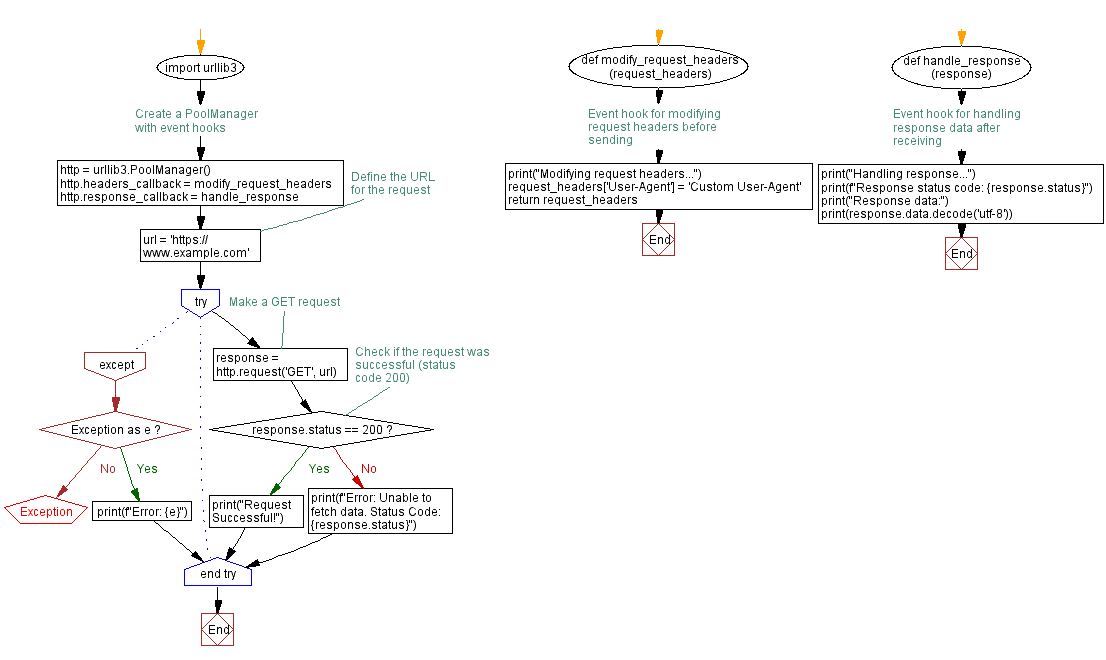
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python Retry Mechanism with incremental timeouts.
Next: Python URL Parsing and Modification for Requests.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.