Python URL Parsing and Modification for Requests
Write a Python program that manually parses a URL, modifies its components, and then reconstruct the URL for a request.
Sample Solution:
Python Code :
import urllib3
from urllib.parse import urlparse, urlunparse
# Original URL
original_url = 'https://www.example.com/path/to/resource?param1=value1¶m2=value2'
# Parse the original URL
parsed_url = urlparse(original_url)
# Modify URL components if needed
parsed_url = parsed_url._replace(path='/new/path', query='new_param=new_value')
# Reconstruct the modified URL
modified_url = urlunparse(parsed_url)
# Create a PoolManager and make a request with the modified URL
http = urllib3.PoolManager()
try:
# Make a GET request with the modified URL
response = http.request('GET', modified_url)
# Check if the request was successful (status code 200)
if response.status == 200:
print("Request Successful!")
print("Response:")
print(response.data.decode('utf-8'))
else:
print(f"Error: Unable to fetch data. Status Code: {response.status}")
except Exception as e:
print(f"Error: {e}")
Sample Output:
Error: Unable to fetch data. Status Code: 404
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Start with an original URL (original_url).
- Parse the original URL using "urlparse()" to get its components.
- Modify the URL components as needed.
- After modifying the components, we reconstruct the URL using "urlunparse()".
- Finally, create a "PoolManager" object, make a GET request with the modified URL, and handle the response.
Flowchart:
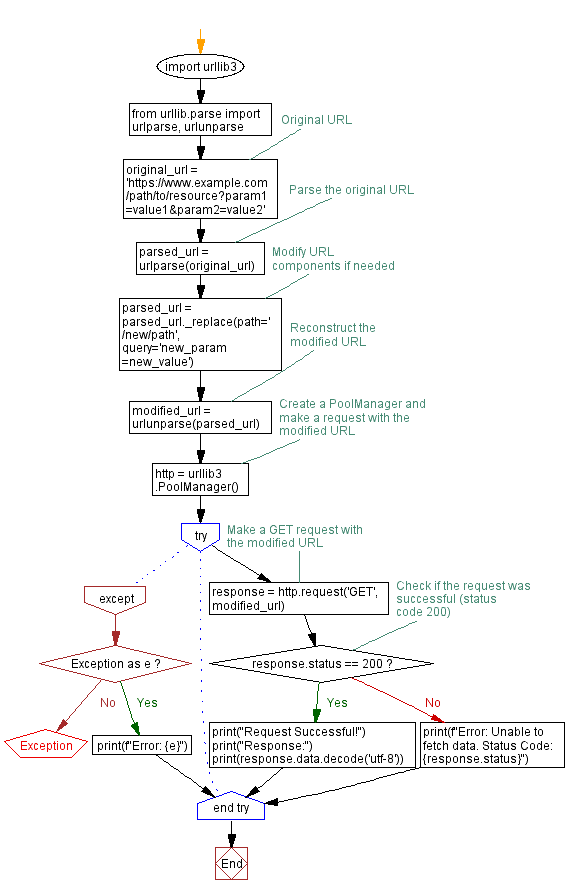
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python Event Hooks for Request and Response Customization.
Next: Python HTTP Request with Manual response decompression.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.