Python HTTP Request with Manual response decompression
Write a Python program that makes a request to a server that sends a compressed response. Manually decompresses the content using the urllib3 function.
Sample Solution:
Python Code :
import urllib3
import gzip
from io import BytesIO
# Create a PoolManager
http = urllib3.PoolManager()
# Define the URL of the server that sends a compressed response
url = 'https://example.com/compressed-data'
try:
# Make a GET request
response = http.request('GET', url)
# Check if the response is compressed (content-encoding header)
if 'gzip' in response.headers.get('content-encoding', ''):
# Decompress the content manually
compressed_data = BytesIO(response.data)
decompressed_data = gzip.GzipFile(fileobj=compressed_data).read()
print("Decompressed Content:")
print(decompressed_data.decode('utf-8'))
else:
print("Response is not compressed.")
except Exception as e:
print(f"Error: {e}")
Sample Output:
Response is not compressed.
Note: If the response is not compressed, it means that the server did not send the response with compression enabled. This could be due to various reasons, such as the server not supporting compression, the client not specifying it can accept compressed responses. In addition, the data being small enough that compression is not beneficial.
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- First create a "PoolManager" object to manage the connection pool.
- Define the URL of the server that sends a compressed response.
- Make a GET request to the server.
- Check if the response is compressed by looking at the "content-encoding" header.
- If the response is compressed, we manually decompress the content using the "gzip" library and print the decompressed content.
- If the response is not compressed, we print a message indicating that the response is not compressed.
Flowchart:
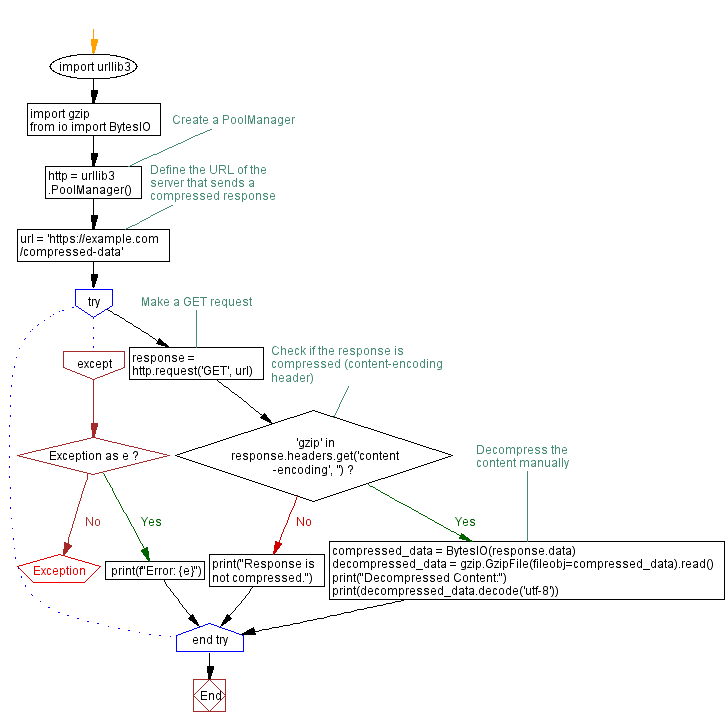
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.