C Exercises: Get the absolute difference between n and 51
C-programming basic algorithm: Exercise-2 with Solution
Write a C program that will take a number as input and find the absolute difference between the input number and 51. If the input number is greater than 51, it will return triple the absolute difference.
C Code:
#include <stdio.h> // Include standard input/output library
int test(int n); // Declare the function 'test' with an integer parameter
int main(void)
{
// Call the function 'test' with argument 53 and print the result
printf("%d", test(53));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with argument 30 and print the result
printf("%d", test(30));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with argument 51 and print the result
printf("%d", test(51));
}
// Function definition for 'test'
int test(int n)
{
const int x = 51; // Declare and initialize constant variable 'x'
if (n > x) // Check if 'n' is greater than 'x'
{
return (n - x) * 3; // Return the result of the expression (n - x) multiplied by 3
}
return x - n; // Return the result of the expression x minus n
}
Sample Output:
6 21 0
Explanation:
int test(int n) { const int x = 51; if (n > x) { return (n - x) * 3; } return x - n; }
The above function takes an integer value n as input and returns the value based on the condition. If n is greater than a constant value x (which is 51 in this case), it returns the result of (n-x)*3, otherwise, it returns the result of x-n.
Time complexity and space complexity:
Time complexity: The time complexity of this function is O(1) since the execution time of this function does not depend on the size of the input n.
Space complexity: The space complexity of this function is also O(1) since the amount of memory used by the function does not depend on the size of the input n.
Pictorial Presentation:
Flowchart:
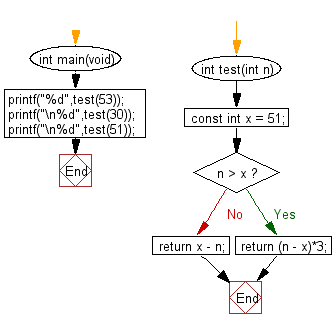
C Programming Code Editor:
Previous: Write a C program to compute the sum of the two given integer values. If the two values are the same, then return triple their sum.
Next: Write a C program to check two given integers, and return true if one of them is 30 or if their sum is 30.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics