Java: Check if a given array contains a subarray with 0 sum
Java Array: Exercise-54 with Solution
Write a Java program to check if a given array contains a subarray with 0 sum.
Example:
Input :
nums1= { 1, 2, -2, 3, 4, 5, 6 }
nums2 = { 1, 2, 3, 4, 5, 6 }
nums3 = { 1, 2, -3, 4, 5, 6 }
Output:
Does the said array contain a subarray with 0 sum: true
Does the said array contain a subarray with 0 sum: false
Does the said array contain a subarray with 0 sum: true
Sample Solution:
Java Code:
// Import the necessary Java utility classes.
import java.util.Set;
import java.util.HashSet;
import java.util.Arrays;
// Define a class named 'solution'.
class solution
{
// A method to find if there exists a subarray with a sum of zero.
public static Boolean find_subarray_sum_zero(int[] nums)
{
// Create a HashSet to store the cumulative sum of elements.
Set set = new HashSet<>();
set.add(0);
int suba_sum = 0;
// Iterate through the elements of the array.
for (int i = 0; i < nums.length; i++)
{
suba_sum += nums[i];
// If the cumulative sum already exists in the set, return true.
if (set.contains(suba_sum)) {
return true;
}
// Add the cumulative sum to the set.
set.add(suba_sum);
}
// If no subarray with a sum of zero is found, return false.
return false;
}
public static void main (String[] args)
{
// Define an array 'nums1'.
int[] nums1 = { 1, 2, -2, 3, 4, 5, 6 };
System.out.println("Original array: " + Arrays.toString(nums1));
// Check if the array contains a subarray with a sum of zero and print the result.
System.out.println("Does the said array contain a subarray with 0 sum: " + find_subarray_sum_zero(nums1));
// Define another array 'nums2'.
int[] nums2 = { 1, 2, 3, 4, 5, 6 };
System.out.println("\nOriginal array: " + Arrays.toString(nums2));
// Check if the array contains a subarray with a sum of zero and print the result.
System.out.println("Does the said array contain a subarray with 0 sum: " + find_subarray_sum_zero(nums2));
// Define yet another array 'nums3'.
int[] nums3 = { 1, 2, -3, 4, 5, 6 };
System.out.println("\nOriginal array: " + Arrays.toString(nums3));
// Check if the array contains a subarray with a sum of zero and print the result.
System.out.println("Does the said array contain a subarray with 0 sum: " + find_subarray_sum_zero(nums3));
}
}
Sample Output:
Original array: [1, 2, -2, 3, 4, 5, 6] Does the said array contain a subarray with 0 sum: true Original array: [1, 2, 3, 4, 5, 6] Does the said array contain a subarray with 0 sum: false Original array: [1, 2, -3, 4, 5, 6] Does the said array contain a subarray with 0 sum: true
Flowchart:
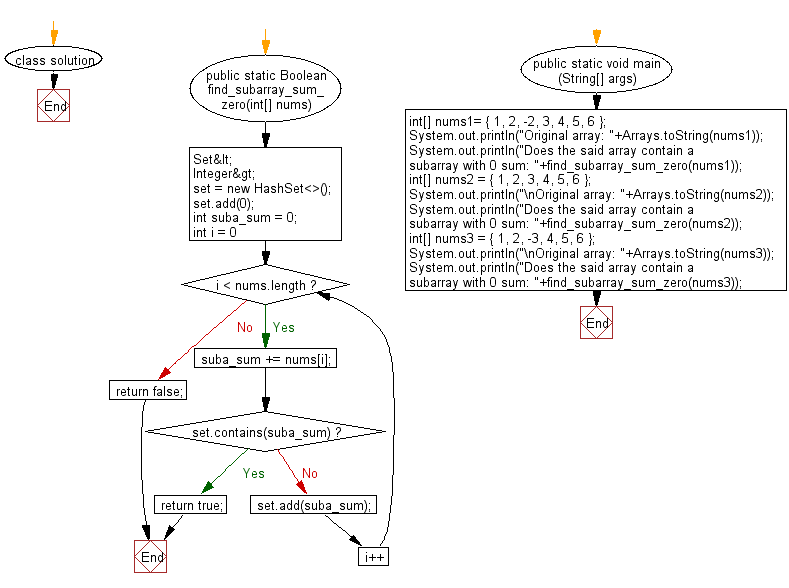
Java Code Editor:
Previous: Write a Java program to replace every element with the next greatest element (from right side) in a given array of integers.
Next: Write a Java program to print all sub-arrays with 0 sum present in a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics