JavaFX GridPane: Simple form creation example
JavaFx Layout Management: Exercise-4 with Solution
Write a JavaFX program that uses a GridPane to create a simple form with labels and text fields.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Simple Form Example");
// Create a GridPane layout
GridPane gridPane = new GridPane();
gridPane.setHgap(10);
gridPane.setVgap(10);
// Create labels and text fields
Label nameLabel = new Label("Name:");
TextField nameField = new TextField();
Label ageLabel = new Label("Age:");
TextField ageField = new TextField();
Label emailLabel = new Label("Email:");
TextField emailField = new TextField();
// Add labels and text fields to the GridPane
gridPane.add(nameLabel, 0, 0);
gridPane.add(nameField, 1, 0);
gridPane.add(ageLabel, 0, 1);
gridPane.add(ageField, 1, 1);
gridPane.add(emailLabel, 0, 2);
gridPane.add(emailField, 1, 2);
// Create the scene and set it in the stage
Scene scene = new Scene(gridPane, 300, 200); // Width and height of the window
primaryStage.setScene(scene);
// Show the window
primaryStage.show();
}
}
In the exercise above, we create a 'GridPane' layout and add labels and text fields to create a simple form. The 'setHgap' and 'setVgap' methods are used to set horizontal and vertical gaps between cells in the grid. You can run this application to see the simple form layout.
Sample Output:
Flowchart:
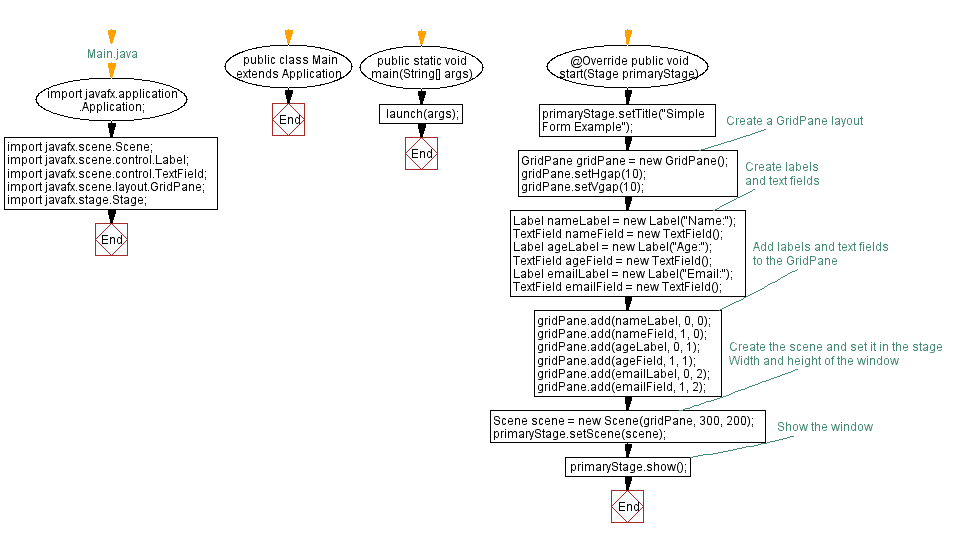
Java Code Editor:
Previous: BorderPane Layout: JavaFX application example.
Next: JavaFX VBox with custom spacing.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics