JavaScript: Test whether a string starts with a specified string
JavaScript String: Exercise-46 with Solution
Write a JavaScript function to test whether a string starts with a specified string.
Test Data:
console.log(startsWith('js string exercises', 'js'));
true
Visual Presentation:
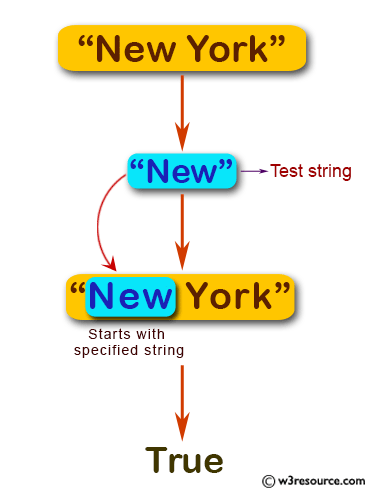
Sample Solution:
JavaScript Code:
// Define a function called startsWith that takes two parameters: input (a string) and string (another string).
function startsWith(input, string) {
// Check if the index of the second string within the first string is 0, indicating that the first string starts with the second string.
return input.indexOf(string) === 0;
}
// Test the startsWith function by calling it with two strings and logging the result to the console.
console.log(startsWith('js string exercises', 'js'));
Output:
true
Explanation:
The above code defines a function named "startsWith()" that checks if a given input string starts with a specified substring. It takes two parameters: 'input', which is the string to be checked, and 'string', which is the substring to check for at the beginning of the input string. Inside the function, it uses the 'indexOf' method to determine the position of the substring within the input string.
If the substring is found at the beginning (position 0), the function returns 'true'; otherwise, it returns 'false'.
Finally, the code tests the "startsWith()" function with a sample input and logs the result to the console.
Flowchart:
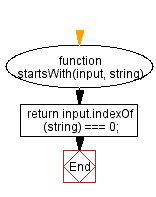
Live Demo:
See the Pen JavaScript Test whether a string starts with a specified string-string-ex-46 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get humanized number with the correct suffix such as 1st, 2nd, 3rd or 4th.
Next: Write a JavaScript function to test whether a string ends with a specified string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics