JavaScript: Test whether a string ends with a specified string
JavaScript String: Exercise-47 with Solution
Write a JavaScript function to test whether a string ends with a specified string.
Test Data:
console.log(endsWith('JS string exercises', 'exercises'));
true
Visual Presentation:
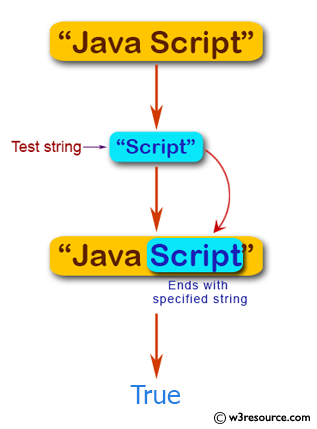
Sample Solution:
JavaScript Code:
// Define a function named endsWith that checks if a given input string ends with a specified substring.
function endsWith(input, string) {
// Calculate the starting index from where to search for the substring in the input string.
var index = input.length - string.length;
// Check if the calculated index is non-negative and if the substring is found in the input string starting from the calculated index.
return index >= 0 && input.indexOf(string, index) > -1;
}
// Test the endsWith function with a sample input and log the result to the console.
console.log(endsWith('JS string exercises', 'exercises'));
Output:
true
Explanation:
In the exercise above,
- The function "endsWith()" takes two parameters: 'input' (the string to be checked) and 'string' (the substring to check for at the end of 'input').
- Index Calculation:
- The variable 'index' is calculated as the starting index from where to search for the substring in the input string. It is calculated as the difference between the length of the input string and the length of the specified substring.
- Check Condition:
- The function returns 'true' if the calculated 'index' is non-negative (ensuring that the substring can potentially exist at that index) and if the 'indexOf' method finds the substring in the input string starting from the calculated 'index'.
- Return Value:
- The function returns 'true' if the input string ends with the specified substring, otherwise it returns 'false'.
- Testing:
- The function is tested by calling it with a sample input ('JS string exercises' as the input string and 'exercises' as the substring), and the result is logged to the console.
Flowchart:
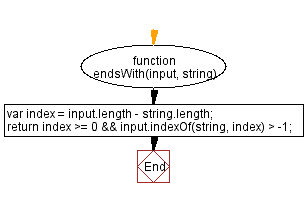
Live Demo:
See the Pen JavaScript Test whether a string ends with a specified string-string-ex-47 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to test whether a string starts with a specified string.
Next: Write a JavaScript function to get the successor of a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics