JavaScript: Get humanized number with the correct suffix
JavaScript String: Exercise-45 with Solution
Humanized Number Suffix
Write a JavaScript function to get a humanized number with the correct suffix such as 1st, 2nd, 3rd or 4th.
Test Data:
console.log(humanize(1));
console.log(humanize(20));
console.log(humanize(302));
"1st"
"20th"
"302nd"
Sample Solution:
JavaScript Code:
// Define a function called humanize that converts a number into a human-readable ordinal representation.
function humanize(number) {
// Check if the last two digits of the number fall between 11 and 13 (inclusive), as these exceptions follow a different ordinal rule.
if (number % 100 >= 11 && number % 100 <= 13)
return number + "th";
// If the last digit of the number is 1, append "st". If it's 2, append "nd". If it's 3, append "rd".
switch (number % 10) {
case 1:
return number + "st";
case 2:
return number + "nd";
case 3:
return number + "rd";
}
// If none of the special cases apply, append "th" to the number.
return number + "th";
}
// Test the humanize function with different numbers and log the results to the console.
console.log(humanize(1)); // Output: "1st"
console.log(humanize(20)); // Output: "20th"
console.log(humanize(302)); // Output: "302nd"
Output:
1st 20th 302nd
Explanation:
The above code defines a function called "humanize()" that converts a given number into a human-readable ordinal representation (e.g., 1st, 2nd, 3rd, 4th, etc.). Here's a breakdown of how it works:
- The function first checks if the last two digits of the number fall between 11 and 13 (inclusive). If they do, it returns the number followed by "th" (e.g., 11th, 12th, 13th). This handles exceptions to the normal ordinal rule.
- If the last digit of the number is 1, 2, or 3, the function appends the appropriate ordinal suffix ("st", "nd", or "rd" respectively) to the number.
- If none of the special cases apply, the function appends "th" to the number.
- The function is then tested with different numbers (1, 20, and 302), and the results are logged to the console using console.log.
Flowchart:
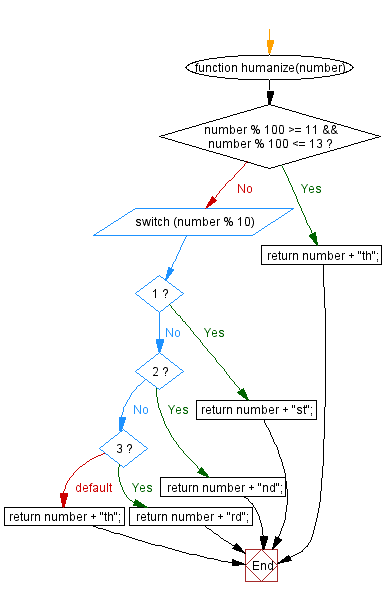
Live Demo:
See the Pen JavaScript Get humanized number with the correct suffix-string-ex-45 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that appends the correct ordinal suffix (st, nd, rd, th) to a number.
- Write a JavaScript function that converts a number to a string and determines its suffix based on its last digit and special cases.
- Write a JavaScript function that handles numbers ending in 11, 12, or 13 correctly by always returning "th".
- Write a JavaScript function that validates the input to ensure it is a positive integer before adding the suffix.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to test whether the character at the provided character index is lower case.
Next: Write a JavaScript function to test whether a string starts with a specified string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.