JavaScript: Test whether the character at the provided character index is lower case
JavaScript String: Exercise-44 with Solution
Is Character Lowercase
Write a JavaScript function to test whether the character at the given (character) index is lower case.
Test Data:
console.log(isLowerCaseAt ('Js STRING EXERCISES', 1));
true
Visual Presentation:
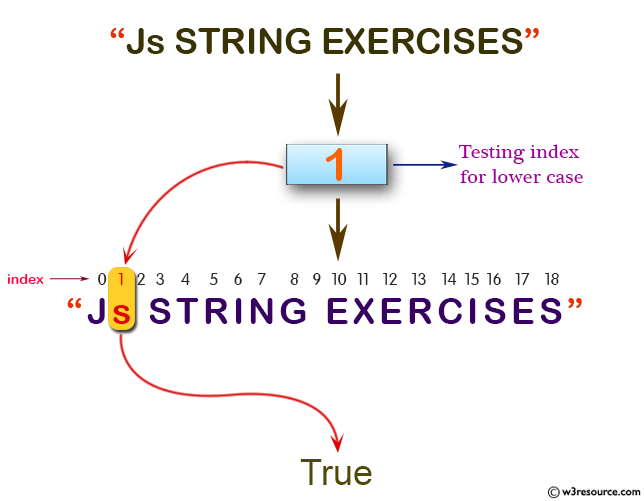
Sample Solution:
JavaScript Code:
// Define a function named isLowerCaseAt which checks if the character at a specific index in a given string is lowercase.
function isLowerCaseAt(str, index) {
// Retrieve the character at the specified index in the input string.
// Convert the character to lowercase and check if it's equal to the original character at that index.
return str.charAt(index).toLowerCase() === str.charAt(index);
}
// Print the result of calling the isLowerCaseAt function with the string 'Js STRING EXERCISES' and index 1.
console.log(isLowerCaseAt('Js STRING EXERCISES', 1));
Output:
true
Explanation:
In the exercise above,
- function isLowerCaseAt(str, index) {: This line declares a function named "isLowerCaseAt()" with two parameters: 'str' (the input string) and 'index' (the index of the character to check).
- return str.charAt(index).toLowerCase() === str.charAt(index);: This line checks if the character at the specified index in the input string, converted to lowercase, is equal to the original character at that index. It returns 'true' if the character is lowercase, and 'false' otherwise.
- console.log(isLowerCaseAt ('Js STRING EXERCISES', 1));: This line calls the "isLowerCaseAt()" function with the input string 'Js STRING EXERCISES' and index 1, and logs the result to the console. It checks if the character at index 1 (the second character) is lowercase.
Flowchart:
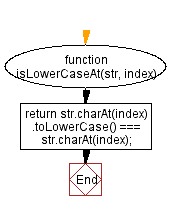
Live Demo:
See the Pen JavaScript Test whether the character at the provided character index is lower case-string-ex-44 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that tests if the character at a specified index of a string is lowercase by comparing it to its lowercase version.
- Write a JavaScript function that checks using charCodeAt() whether a letter falls within the lowercase ASCII range.
- Write a JavaScript function that validates the input and returns false if the index is invalid.
- Write a JavaScript function that accounts for non-letter characters and returns false if the character is not alphabetic.
Go to:
PREV : Is Character Uppercase.
NEXT : Humanized Number Suffix.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.