JavaScript: Test whether the character at the provided character index is upper case
JavaScript String: Exercise-43 with Solution
Is Character Uppercase
Write a JavaScript function to test whether the character at the index provided is upper case.
Test Data:
console.log(isUpperCaseAt('Js STRING EXERCISES', 1));
false
Visual Presentation:
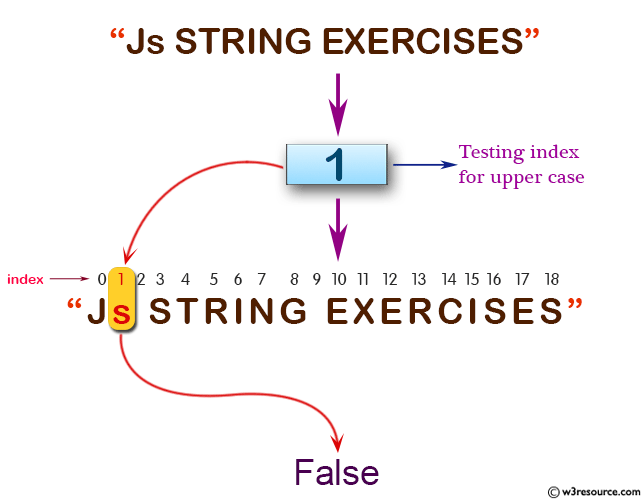
Sample Solution:
JavaScript Code:
// Function to check if the character at a specified index in a string is uppercase
function isUpperCaseAt(str, index) {
// Check if the character at the specified index, converted to uppercase,
// is equal to the character at the specified index
return str.charAt(index).toUpperCase() === str.charAt(index);
}
// Log the result of calling the isUpperCaseAt function with the input string 'Js STRING EXERCISES' and index 1
console.log(isUpperCaseAt('Js STRING EXERCISES', 1));
Output:
false
Explanation:
In the exercise above,
- The function "isUpperCaseAt()" takes two parameters: 'str' (the input string) and 'index' (the index of the character to check).
- Inside the function, it uses the "charAt()" method to retrieve the character at the specified index in the string.
- It then uses the "toUpperCase()" method to convert the character to uppercase.
- Finally, it compares this uppercase character to the original character at the specified index. If they are equal, it means the character at that index was originally uppercase.
- The function returns 'true' if the character at the specified index is uppercase, and 'false' otherwise.
Flowchart:
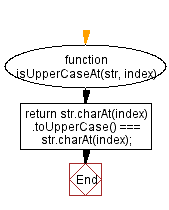
Live Demo:
See the Pen JavaScript Test whether the character at the provided character index is upper case-string-ex-43 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if the character at a specified index of a string is uppercase using charCodeAt().
- Write a JavaScript function that compares the character at a given index with its uppercase version and returns a boolean.
- Write a JavaScript function that validates the input string and index, returning an error if the index is out of range.
- Write a JavaScript function that handles special characters and only evaluates alphabetical characters for uppercase.
Go to:
PREV : Uncapitalize All Words.
NEXT : Is Character Lowercase.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.