JavaScript: Uncapitalize each word in the string
JavaScript String: Exercise-42 with Solution
Uncapitalize All Words
Write a JavaScript function to uncapitalize each word in the string.
Test Data:
console.log(unCapitalizeWords('JS STRING EXERCISES'));
"js string exercises"
Visual Presentation:
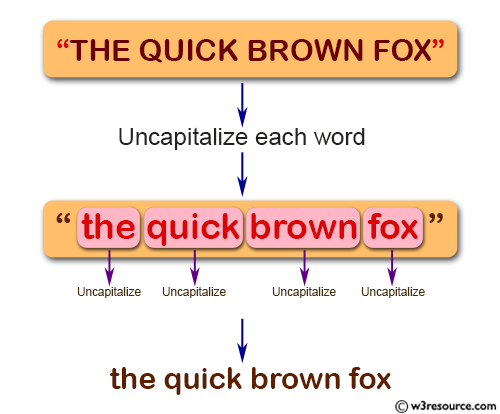
Sample Solution:
JavaScript Code:
// Define a function called unCapitalizeWords taking a string parameter str
function unCapitalizeWords(str) {
// Use the replace method with a regular expression /\w\S*/g to match each word in the string
// For each matched word, execute a callback function
return str.replace(/\w\S*/g, function(txt) {
// In the callback function, convert the first character of each word to lowercase
// and keep the rest of the word unchanged
return txt.substr(0).toLowerCase();
});
}
// Call the unCapitalizeWords function with the input string 'JS STRING EXERCISES'
// and print the resulting string to the console
console.log(unCapitalizeWords('JS STRING EXERCISES'));
Output:
js string exercises
Explanation:
The above code defines a function called "unCapitalizeWords()" that takes a string 'str' as input. The function uses the "replace()" method with a regular expression '\w\S*' to match each word in the string.
For each matched word, a callback function is executed. This converts the first character of the word to lowercase while keeping the rest unchanged.
Finally, the function returns the modified string. The console.log statement calls the unCapitalizeWords function with the input string 'JS STRING EXERCISES' and prints the resulting string to the console.
Flowchart:
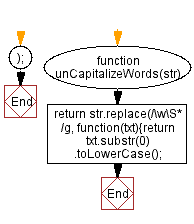
Live Demo:
See the Pen JavaScript Uncapitalize each word in the string-string-ex-42 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts an entire string to lowercase.
- Write a JavaScript function that iterates over each character and converts uppercase letters to lowercase without using toLowerCase().
- Write a JavaScript function that accepts a string and returns the string uncapitalized using built-in methods.
- Write a JavaScript function that validates the input and ensures that non-letter characters remain unchanged.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to capitalize each word in the string.
Next: Write a JavaScript function to test whether the character at the provided (character) index is upper case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.