PHP Array Exercises : Identify the email addresses which are not unique
PHP Array: Exercise-41 with Solution
Write a PHP program to identify the email addresses which are not unique.
Sample Solution:
PHP Code:
<?php
// Define a function to find non-unique values in an array
function array_not_unique($my_array) {
// Initialize an array to store duplicate values
$same = array();
// Perform a natural case-insensitive sort on the array
natcasesort($my_array);
// Reset the array pointer to the beginning
reset($my_array);
// Initialize variables to track the previous key and value
$old_key = NULL;
$old_value = NULL;
// Loop through the sorted array to find duplicate values
foreach ($my_array as $key => $value) {
// Skip NULL values
if ($value === NULL) { continue; }
// Check if the current value is the same as the previous value
if ($old_value == $value) {
// Store the duplicate values and their corresponding keys
$same[$old_key] = $old_value;
$same[$key] = $value;
}
// Update the variables with the current key and value
$old_value = $value;
$old_key = $key;
}
// Return the array containing non-unique values
return $same;
}
// Create a test array with duplicate and non-duplicate values
$test_array = array();
$test_array[1] = '[email protected]';
$test_array[2] = '[email protected]';
$test_array[3] = '[email protected]';
$test_array[4] = '[email protected]';
// Call the function and display the result
print_r(array_not_unique($test_array));
?>
Output:
Array ( [1] => [email protected] [3] => [email protected] )
Flowchart:
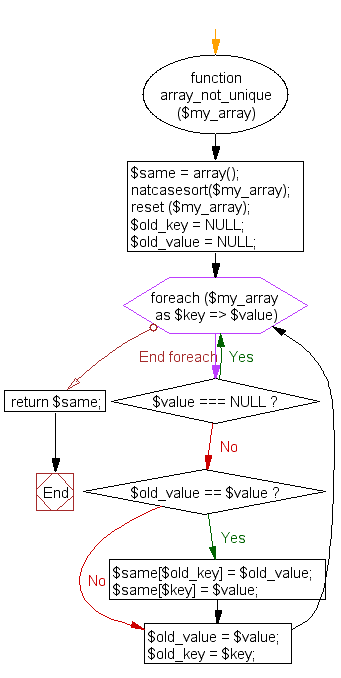
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to get a sorted array without preserving the keys.
Next: Write a PHP function to find unique values from multidimensional arrays and flatten them in 0 depth.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics