PHP Array Exercises : Find unique values from multidimensional array and flatten them in zero depth
42. Unique Flattened Values from Multidimensional Arrays
Write a PHP function to find unique values from multidimensional arrays and flatten them in 0 depth.
Sample Solution:
PHP Code:
<?php
// Define a function to flatten a multidimensional array
function array_flat($my_array)
{
// Initialize an array to store flattened values
$fa = array();
// Initialize a variable to track the recursion depth
$l = 0;
// Loop through the input array
foreach($my_array as $k => $v )
{
// Check if the current value is not an array
if( !is_array( $v ) )
{
// Add the non-array value to the flattened array
$fa[] = $v;
// Continue to the next iteration
continue;
}
// Increment the recursion depth
$l++;
// Recursively flatten the nested array
$fa = array_flat( $v, $fa, $l );
// Decrement the recursion depth
$l--;
}
// Check if the recursion depth is back to the top level
if( $l == 0 )
// Remove duplicates and re-index the array
$fa = array_values( array_unique( $fa ) );
// Return the flattened array
return $fa;
}
// Create a test multidimensional array
$tmp = array( 'a' => array( -1, -2, 0, 2, 3 ), 'b' => array( 'c' => array( -1, 0, 2, 0, 3 ) ) );
// Call the function and display the result
print_r(array_flat($tmp));
?>
Output:
Array ( [0] => -1 [1] => 0 [2] => 2 [3] => 3 )
Flowchart:
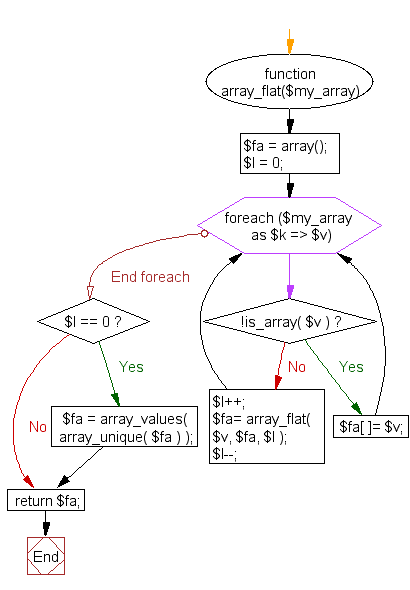
For more Practice: Solve these Related Problems:
- Write a PHP function to recursively traverse a multidimensional array and return a flattened array of unique values.
- Write a PHP script to combine multiple nested arrays into one flat array while filtering duplicate entries.
- Write a PHP program to flatten a multidimensional array and remove duplicates using a custom iteration approach.
- Write a PHP function to merge nested arrays and then apply array_unique() to the flat result and output it.
Go to:
PREV : Identify Non-Unique Email Addresses.
NEXT : Merge Two Comma-Separated Lists with Unique Values.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.