PHP Array Exercises : Sorted array without preserving the keys
40. Sorted Array Without Preserving Keys
Write a PHP program to get a sorted array without preserving the keys.
Sample Solution:
PHP Code:
<?php
// Define an array with duplicate and non-duplicate values
$my_array = array("red", "black", "green", "black", "white", "yellow");
// Use array_unique to remove duplicate values and preserve keys
$sorted_unique_array = array_values(array_unique($my_array));
// Display the resulting array containing unique values
print_r($sorted_unique_array);
?>
Output:
Array ( [0] => Red [1] => Green [2] => White [3] => Black ) Array ( [0] => 100 [1] => 200 [2] => -10 [3] => 0 )
Flowchart:
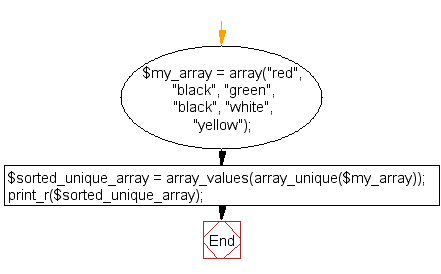
For more Practice: Solve these Related Problems:
- Write a PHP script to sort an array of integers in ascending order and then reindex the array using array_values().
- Write a PHP function to perform a natural sort on an array and output the result as a zero-indexed array.
- Write a PHP program to sort an associative array by value, then convert it to a numerically indexed array for output.
- Write a PHP script to implement sorting on an array while stripping away the original keys and displaying only the values.
Go to:
PREV : Remove Duplicate Values from Array.
NEXT : Identify Non-Unique Email Addresses.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.