NumPy: Repeat elements of an array
NumPy: Array Object Exercise-26 with Solution
Write a NumPy program to repeat array elements.
Pictorial Presentation:
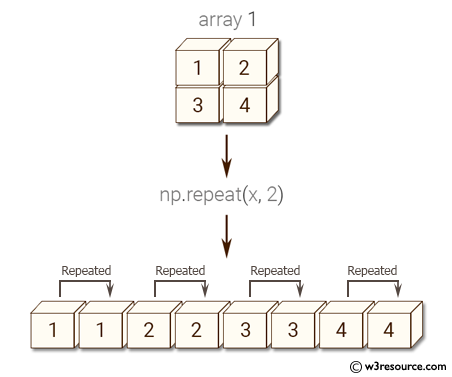
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Repeating the value 3, four times and creating a NumPy array
x = np.repeat(3, 4)
print(x)
# Creating a NumPy array
x = np.array([[1, 2], [3, 4]])
# Repeating each element in the array twice
print(np.repeat(x, 2))
Sample Output:
[3 3 3 3] [1 1 2 2 3 3 4 4]
Explanation:
In the above code -
x = np.repeat(3, 4): The np.repeat function repeats the value 3 four times, creating a new NumPy array: [3, 3, 3, 3].
x = np.array([[1, 2], [3, 4]]): A 2x2 NumPy array x is created with elements [1, 2] in the first row and [3, 4] in the second row.
print(np.repeat(x, 2)): The np.repeat function is called again, this time with the 2D NumPy array x. By default, the elements in the input array are flattened, and then each element is repeated twice. The resulting 1D array is [1, 1, 2, 2, 3, 3, 4, 4].
The final output is printed: [1, 1, 2, 2, 3, 3, 4, 4].
Python-Numpy Code Editor:
Previous: Write a NumPy program to construct an array by repeating.
Next: Write a NumPy program to find the indices of the maximum and minimum values along the given axis of an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics