NumPy: Find the indices of the maximum and minimum values along the given axis of an array
Indices of Max/Min Along Axis
Write a NumPy program to find the indices of the maximum and minimum values along the given axis of an array.
Pictorial Presentation:
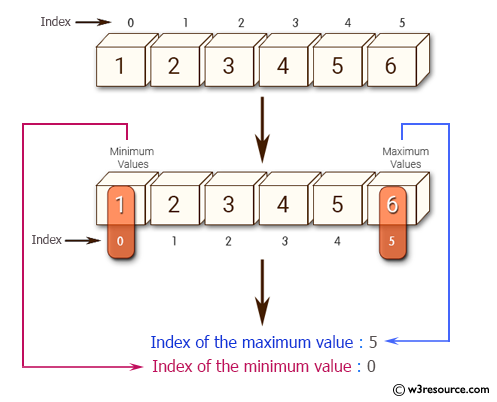
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array
x = np.array([1, 2, 3, 4, 5, 6])
# Displaying the original array
print("Original array: ", x)
# Finding the index of the maximum value in the array
print("Maximum Values: ", np.argmax(x))
# Finding the index of the minimum value in the array
print("Minimum Values: ", np.argmin(x))
Sample Output:
Original array: [1 2 3 4 5 6] Maximum Values: 5 Minimum Values: 0
Explanation:
In the above code -
x = np.array([1, 2, 3, 4, 5, 6]): A 1D NumPy array x is created with elements [1, 2, 3, 4, 5, 6].
print("Maximum Values: ", np.argmax(x)): The np.argmax function finds the index of the maximum value in the array x. In this case, the maximum value is 6, and its index is 5.
The output is: Maximum Values: 5.
print("Minimum Values: ", np.argmin(x)): The np.argmin function finds the index of the minimum value in the array x. In this case, the minimum value is 1, and its index is 0. The output is: Minimum Values: 0.
For more Practice: Solve these Related Problems:
- Find the indices of the maximum and minimum elements along a given axis using np.argmax and np.argmin.
- Create a function that returns both max and min indices from a multi-dimensional array.
- Test the index-finding function on an array with duplicate maximum or minimum values to observe its behavior.
- Compare the indices obtained by np.argmax with those computed by manual iteration over array slices.
Go to:
PREV : Repeat Each Array Element
NEXT : Compare Two Arrays Element-Wise
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.