Python PyQt drag and drop labels example
Python PyQt Event Handling: Exercise-6 with Solution
Write a Python program that allows users to drag and drop two labels within a window using PyQt.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QLabel Class: The QLabel widget provides a text or image display.
Qt module: PyQt5 is a set of Python bindings for the Qt application framework. It allows us to use Qt, a popular C++ framework, to create graphical user interfaces (GUIs) in Python.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel
from PyQt5.QtCore import Qt
class DraggableLabel(QLabel):
def __init__(self, text, parent=None):
super().__init__(text, parent)
self.setAcceptDrops(True)
self.mousePressPos = None
self.mouseMovePos = None
def mousePressEvent(self, event):
if event.button() == Qt.LeftButton:
self.mousePressPos = event.globalPos()
self.mouseMovePos = event.globalPos()
super().mousePressEvent(event)
def mouseMoveEvent(self, event):
if event.buttons() == Qt.LeftButton:
if self.mousePressPos:
delta = event.globalPos() - self.mouseMovePos
self.move(self.x() + delta.x(), self.y() + delta.y())
self.mouseMovePos = event.globalPos()
super().mouseMoveEvent(event)
def mouseReleaseEvent(self, event):
if event.button() == Qt.LeftButton:
self.mousePressPos = None
super().mouseReleaseEvent(event)
class DragAndDropApp(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Drag and Drop Example")
self.setGeometry(100, 100, 400, 400)
label1 = DraggableLabel("Drag this label!", self)
label1.setGeometry(50, 50, 100, 30)
label2 = DraggableLabel("Drag this label too!", self)
label2.setGeometry(150, 150, 120, 30)
def main():
app = QApplication(sys.argv)
window = DragAndDropApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary PyQt5 modules.
- Create a custom "DraggableLabel" class that inherits from "QLabel".
- Override the "mousePressEvent", "mouseMoveEvent", and "mouseReleaseEvent" methods to handle mouse interactions. When the left mouse button is pressed, we record the initial mouse position and update the label's position as the mouse is moved.
- Create two draggable labels and add them to the main window.
- When you run the program, you can drag and drop labels within a window
Output:
Flowchart:
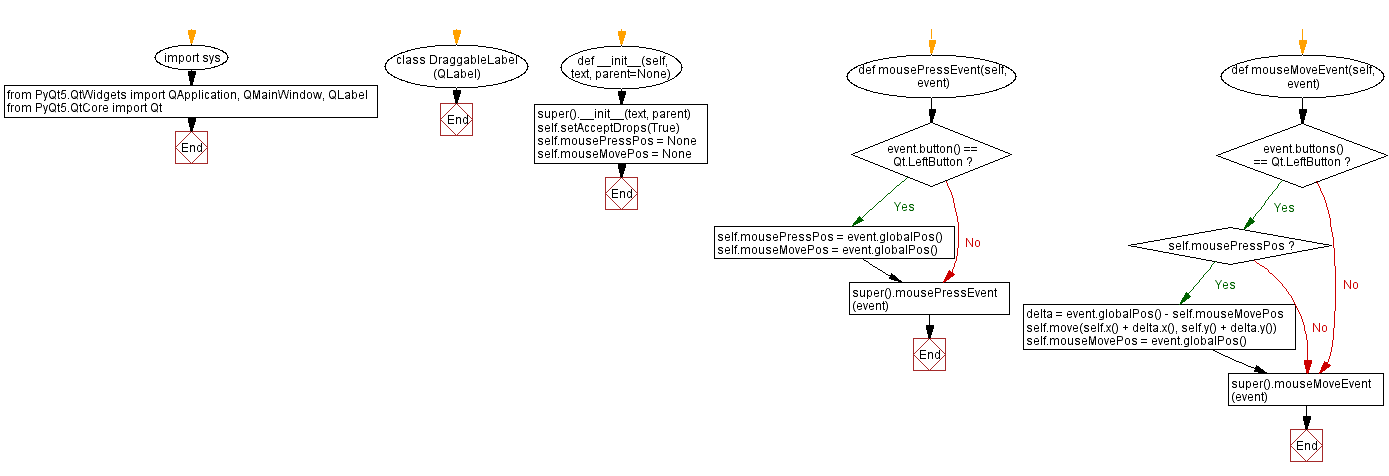
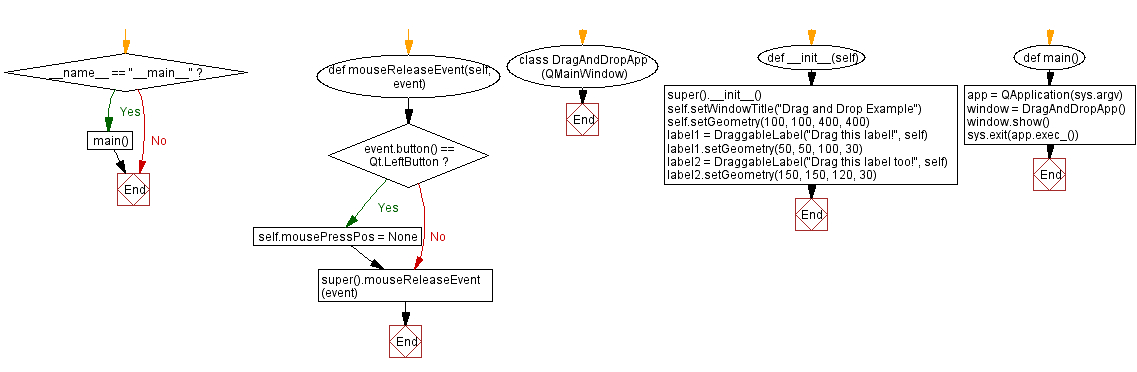
Python Code Editor:
Previous: Python PyQt custom widget with keyboard shortcuts.
Next: Python PyQt mouse tracking example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics