C Exercises: Display the lower triangular of a given matrix
C Array: Exercise-26 with Solution
Write a program in C to print or display the lower triangular of a given matrix.
Visual Presentation:
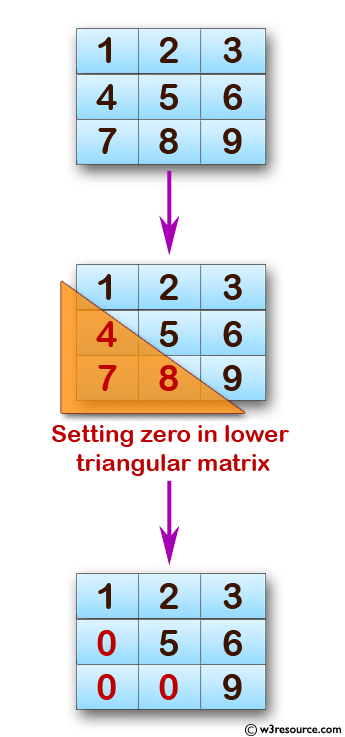
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int arr1[10][10], i, j, n;
float determinant = 0;
// Display the purpose of the program
printf("\n\nDisplay the lower triangular of a given matrix :\n");
printf("----------------------------------------------------\n");
// Input the size of the square matrix
printf("Input the size of the square matrix : ");
scanf("%d", &n);
// Input elements into the matrix
printf("Input elements in the matrix :\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &arr1[i][j]);
}
}
// Display the original matrix
printf("The matrix is :\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
printf("% 4d", arr1[i][j]);
}
printf("\n");
}
// Display lower triangular matrix by setting upper triangular elements to zero
printf("\nSetting zero in lower triangular matrix\n");
for (i = 0; i < n; i++) {
printf("\n");
for (j = 0; j < n; j++) {
if (i <= j) {
printf("% 4d", arr1[i][j]); // Print the original elements if i <= j (lower triangular)
} else {
printf("% 4d", 0); // Print zero if i > j (upper triangular)
}
}
}
printf("\n\n");
return 0;
}
Sample Output:
Display the lower triangular of a given matrix : ---------------------------------------------------- Input the size of the square matrix : 3 Input elements in the first matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [0],[2] : 3 element - [1],[0] : 4 element - [1],[1] : 5 element - [1],[2] : 6 element - [2],[0] : 7 element - [2],[1] : 8 element - [2],[2] : 9 The matrix is : 1 2 3 4 5 6 7 8 9 Setting zero in lower triangular matrix 1 2 3 0 5 6 0 0 9
Flowchart:
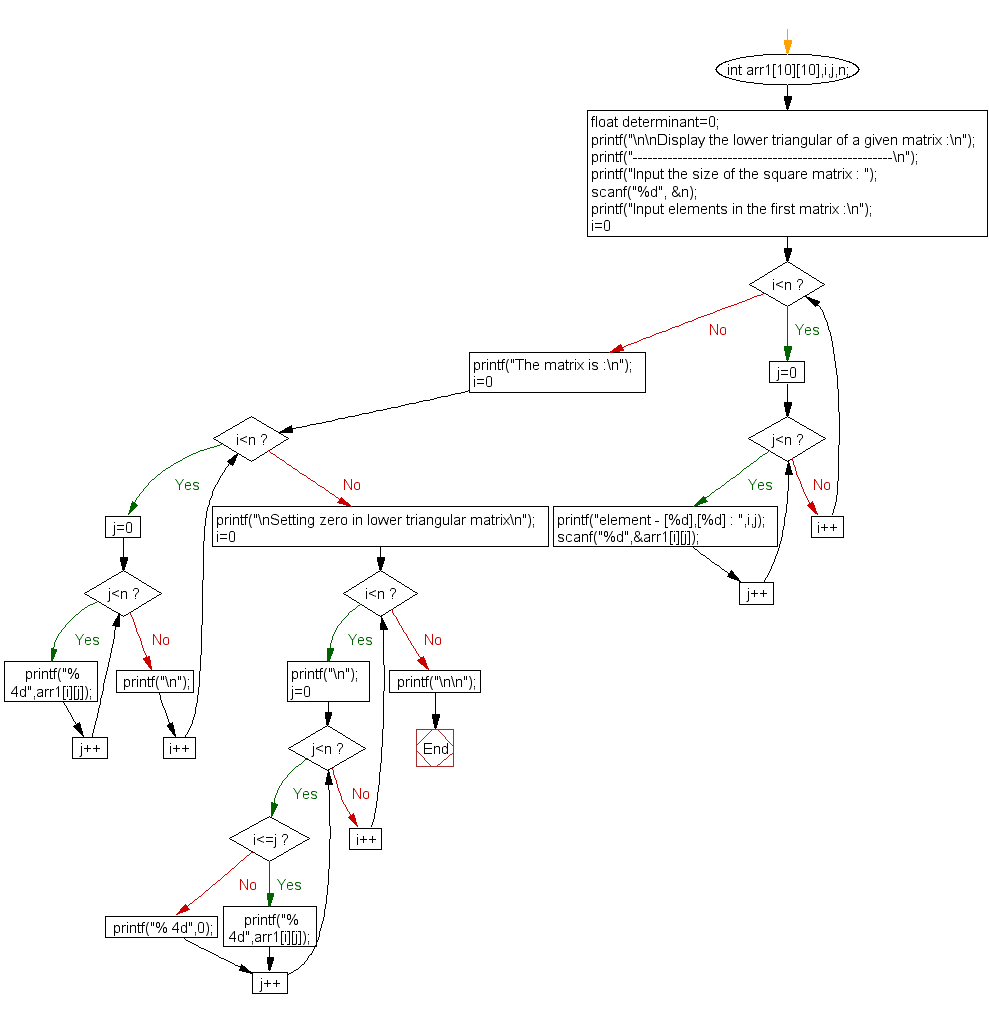
C Programming Code Editor:
Previous: Write a program in C to find the sum of rows an columns of a Matrix.
Next: Write a program in C to print or display upper triangular matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics