C Exercises: Find the sum of rows an columns of a Matrix
25. Sum of Rows & Columns
Write a program in C to find the sum of rows and columns of a matrix.
The task is to write a C program that computes the sum of rows and columns of a square matrix. The program prompts the user to input the size of the matrix and its elements, calculates the sum of each row and column, and displays the original matrix followed by the sums of its rows and columns as output.
Visual Presentation:
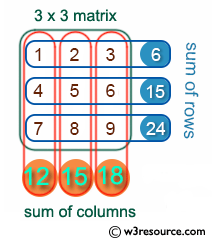
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int i, j, k, arr1[10][10], rsum[10], csum[10], n;
// Display the purpose of the program
printf("\n\nFind the sum of rows and columns of a Matrix:\n");
printf("-------------------------------------------\n");
// Input the size of the square matrix
printf("Input the size of the square matrix : ");
scanf("%d", &n);
// Input elements into the matrix
printf("Input elements in the matrix :\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &arr1[i][j]);
}
}
// Display the matrix
printf("The matrix is :\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
printf("% 4d", arr1[i][j]);
}
printf("\n");
}
// Calculate the sum of rows
for (i = 0; i < n; i++) {
rsum[i] = 0;
for (j = 0; j < n; j++) {
rsum[i] = rsum[i] + arr1[i][j];
}
}
// Calculate the sum of columns
for (i = 0; i < n; i++) {
csum[i] = 0;
for (j = 0; j < n; j++) {
csum[i] = csum[i] + arr1[j][i];
}
}
// Display the matrix with row sums
printf("The sum of rows and columns of the matrix is :\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
printf("% 4d", arr1[i][j]);
}
printf("% 8d", rsum[i]); // Print the sum of each row
printf("\n");
}
// Display the column sums
printf("\n");
for (j = 0; j < n; j++) {
printf("% 4d", csum[j]); // Print the sum of each column
}
printf("\n\n");
return 0;
}
Sample Output:
Find the sum of rows an columns of a Matrix: ------------------------------------------- Input the size of the square matrix : 2 Input elements in the first matrix : element - [0],[0] : 5 element - [0],[1] : 6 element - [1],[0] : 7 element - [1],[1] : 8 The matrix is : 5 6 7 8 The sum or rows and columns of the matrix is : 5 6 11 7 8 15 12 14
Flowchart:
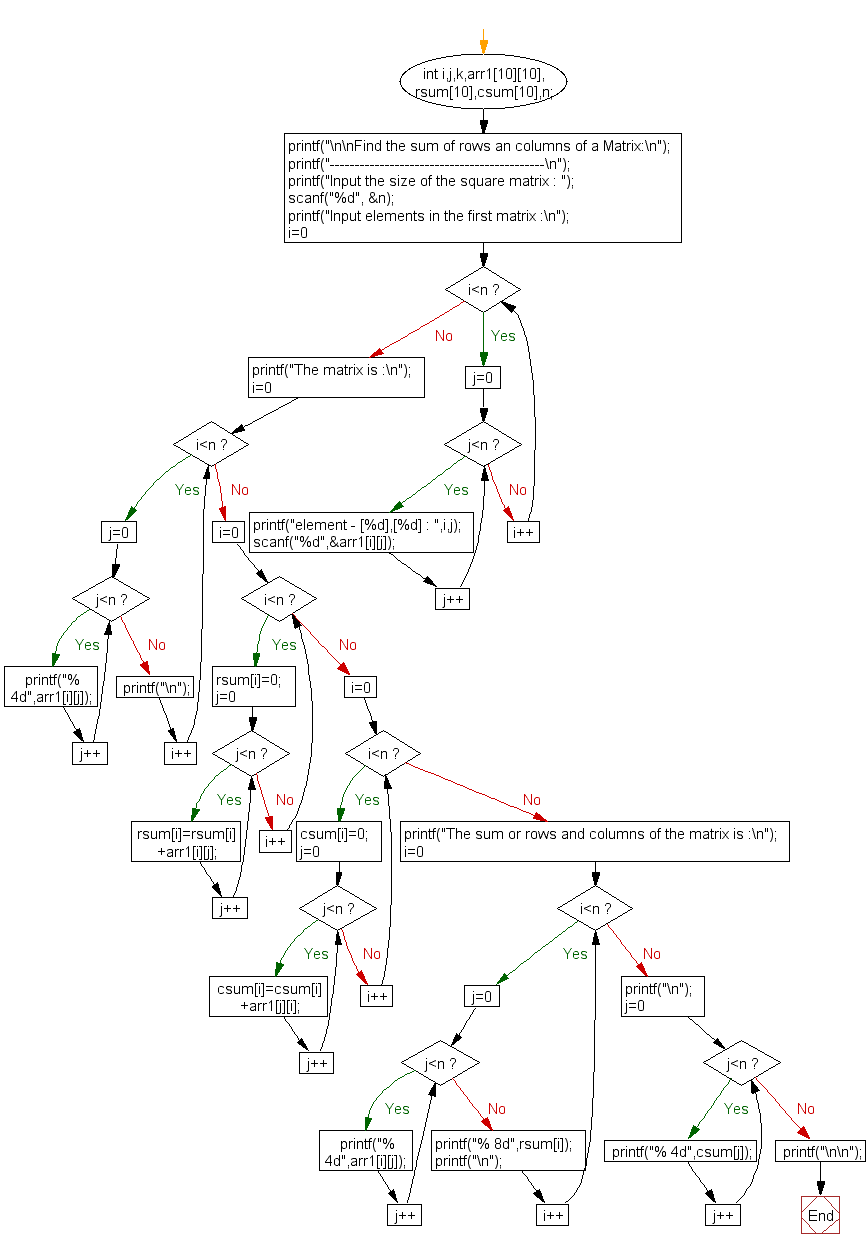
For more Practice: Solve these Related Problems:
- Write a C program to compute the sum of each row and each column in a matrix and display them alongside the matrix.
- Write a C program to calculate the row and column sums of a matrix and then determine the overall total sum.
- Write a C program to compute and print the row-wise average and column-wise average of a matrix.
- Write a C program to calculate row and column sums using dynamic memory allocation for matrices.
C Programming Code Editor:
Previous: Write a program in C to find sum of left diagonals of a matrix.
Next: Write a program in C to print or display the lower triangular of a given matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.