C Exercises: Display the upper triangular of a given matrix
27. Upper Triangular Matrix Display
Write a program in C to print or display an upper triangular matrix.
The task is to write a C program that prints the upper triangular matrix of a given square matrix. The program prompts the user to input the size of the matrix and its elements, displays the original matrix, then modifies the matrix to show only its upper triangular part (where elements below the main diagonal are set to zero) and prints this modified matrix as output.
Visual Presentation:
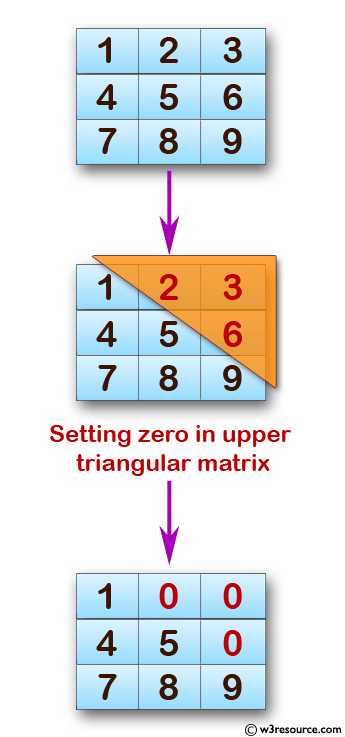
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int arr1[10][10], i, j, n;
float determinant = 0;
// Display the purpose of the program
printf("\n\nDisplay the upper triangular of a given matrix :\n");
printf("----------------------------------------------\n");
// Input the size of the square matrix
printf("Input the size of the square matrix : ");
scanf("%d", &n);
// Input elements into the matrix
printf("Input elements in the matrix :\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &arr1[i][j]);
}
}
// Display the original matrix
printf("The matrix is :\n");
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
printf("% 4d", arr1[i][j]);
}
printf("\n");
}
// Display upper triangular matrix by setting lower triangular elements to zero
printf("\nSetting zero in upper triangular matrix\n");
for (i = 0; i < n; i++) {
printf("\n");
for (j = 0; j < n; j++) {
if (i >= j) {
printf("% 4d", arr1[i][j]); // Print the original elements if i >= j (upper triangular)
} else {
printf("% 4d", 0); // Print zero if i < j (lower triangular)
}
}
}
printf("\n\n");
return 0;
}
Sample Output:
Display the upper triangular of a given matrix : ---------------------------------------------- Input the size of the square matrix : 3 Input elements in the first matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [0],[2] : 3 element - [1],[0] : 4 element - [1],[1] : 5 element - [1],[2] : 6 element - [2],[0] : 7 element - [2],[1] : 8 element - [2],[2] : 9 The matrix is : 1 2 3 4 5 6 7 8 9 Setting zero in upper triangular matrix 1 0 0 4 5 0 7 8 9
Flowchart:
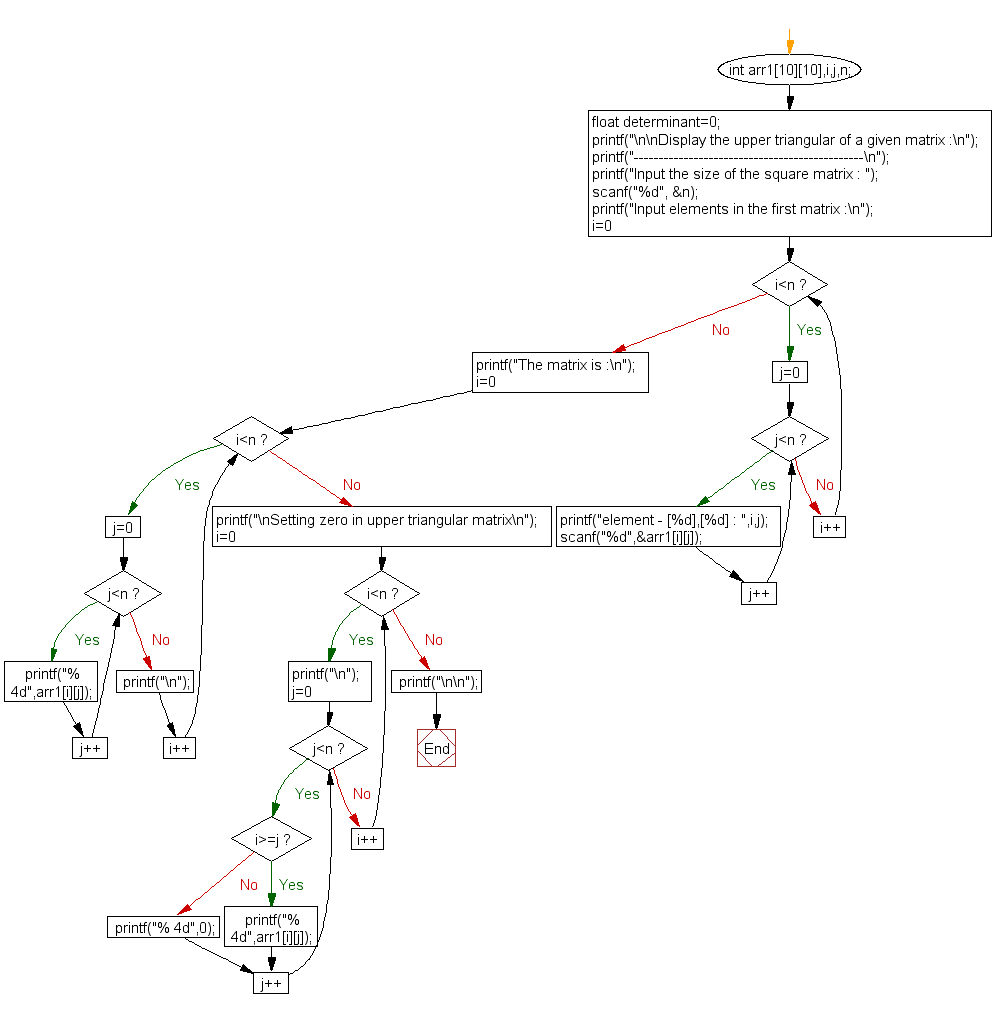
For more Practice: Solve these Related Problems:
- Write a C program to display the upper triangular portion of a matrix and set non-upper elements to zero.
- Write a C program to extract the upper triangular matrix and compute the product of its non-zero elements.
- Write a C program to display the upper triangular matrix using recursion.
- Write a C program to display the upper triangular matrix and then compute the sum of each column.
C Programming Code Editor:
Previous: Write a program in C to print or display the lower triangular of a given matrix.
Next: Write a program in C to calculate determinant of a 3 x 3 matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.