C Exercises: Calculate the determinant of a 3 x 3 matrix
28. 3x3 Matrix Determinant
Write a program in C to calculate the determinant of a 3 x 3 matrix.
The task is to write a C program that calculates the determinant of a 3x3 matrix. The program prompts the user to input the elements of the matrix, displays the original matrix, computes its determinant using the formula for 3x3 matrices, and then prints the calculated determinant as the output.
Visual Presentation:
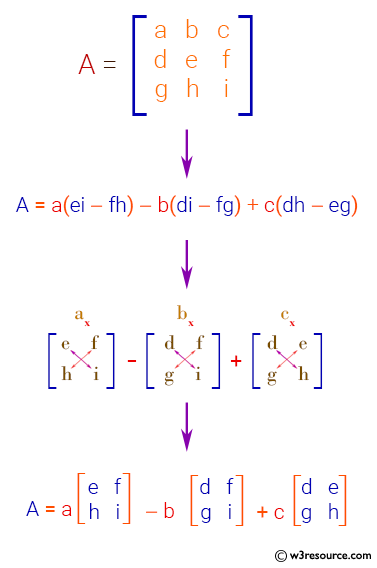
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int arr1[10][10], i, j, n;
int det = 0;
// Display the purpose of the program
printf("\n\nCalculate the determinant of a 3 x 3 matrix :\n");
printf("-------------------------------------------------\n");
// Input elements into the matrix
printf("Input elements in the matrix :\n");
for (i = 0; i < 3; i++) {
for (j = 0; j < 3; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &arr1[i][j]);
}
}
// Display the matrix
printf("The matrix is :\n");
for (i = 0; i < 3; i++) {
for (j = 0; j < 3; j++) {
printf("% 4d", arr1[i][j]);
}
printf("\n");
}
// Calculate the determinant of the 3x3 matrix
for (i = 0; i < 3; i++) {
det = det + (arr1[0][i] * (arr1[1][(i + 1) % 3] * arr1[2][(i + 2) % 3] - arr1[1][(i + 2) % 3] * arr1[2][(i + 1) % 3]));
}
// Display the determinant of the matrix
printf("\nThe Determinant of the matrix is: %d\n\n", det);
return 0;
}
Sample Output:
Calculate the determinant of a 3 x 3 matrix : ------------------------------------------------- Input elements in the first matrix : element - [0],[0] : 1 element - [0],[1] : 0 element - [0],[2] : -1 element - [1],[0] : 0 element - [1],[1] : 0 element - [1],[2] : 1 element - [2],[0] : -1 element - [2],[1] : -1 element - [2],[2] : 0 The matrix is : 1 0 -1 0 0 1 -1 -1 0 The Determinant of the matrix is: 1
Flowchart:
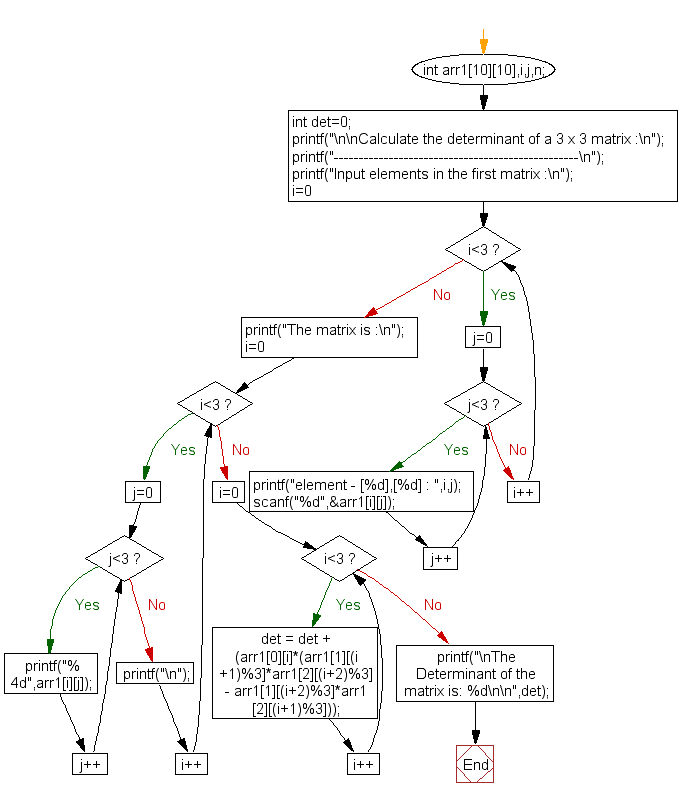
For more Practice: Solve these Related Problems:
- Write a C program to calculate the determinant of a 3x3 matrix using Sarrus’ rule.
- Write a C program to compute the determinant of a 3x3 matrix recursively.
- Write a C program to calculate the determinant and then check if the matrix is invertible.
- Write a C program to compute the determinant of a 3x3 matrix and then solve a system of linear equations.
C Programming Code Editor:
Previous: Write a program in C to print or display upper triangular matrix.
Next: Write a program in C to accept a matrix and determine whether it is a sparse matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.