C Exercises: Determine whether a matrix is a sparse matrix
29. Sparse Matrix Check
Write a program in C to accept a matrix and determine whether it is a sparse matrix.
A sparse martix is matrix which has more zero elements than nonzero elements. The task is to write a C program that accepts a matrix and determines whether it is a sparse matrix. A sparse matrix is one where most of the elements are zero. The program prompts the user for the matrix dimensions and its elements, counts the number of zeros, and then checks if the number of zeros is greater than half the total number of elements to determine and print if the matrix is sparse.
Visual Presentation:
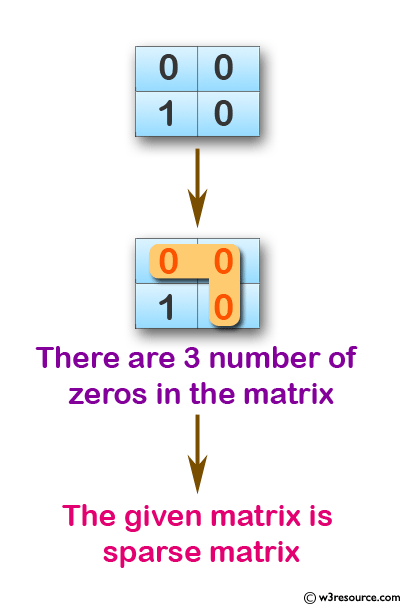
Sample Solution:
C Code:
#include <stdio.h>
/* A sparse matrix is a matrix that has more zero elements than nonzero elements */
int main() {
static int arr1[10][10];
int i, j, r, c;
int ctr = 0;
// Display the purpose of the program
printf("\n\nDetermine whether a matrix is a sparse matrix :\n");
printf("----------------------------------------------------\n");
// Input the number of rows of the matrix
printf("Input the number of rows of the matrix : ");
scanf("%d", &r);
// Input the number of columns of the matrix
printf("Input the number of columns of the matrix : ");
scanf("%d", &c);
// Input elements into the matrix and count zeros
printf("Input elements in the matrix :\n");
for (i = 0; i < r; i++) {
for (j = 0; j < c; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &arr1[i][j]);
if (arr1[i][j] == 0) {
++ctr; // Increment the counter for zero elements
}
}
}
// Check if zeros are more than half of total elements to determine sparsity
if (ctr > ((r * c) / 2)) {
printf("The given matrix is a sparse matrix.\n");
} else {
printf("The given matrix is not a sparse matrix.\n");
}
printf("There are %d number of zeros in the matrix.\n\n", ctr);
return 0;
}
Sample Output:
Determine whether a matrix is a sparse matrix : ---------------------------------------------------- Input the number of rows of the matrix : 2 Input the number of columns of the matrix : 2 Input elements in the first matrix : element - [0],[0] : 0 element - [0],[1] : 0 element - [1],[0] : 1 element - [1],[1] : 0 The given matrix is sparse matrix. There are 3 number of zeros in the matrix.
Flowchart:
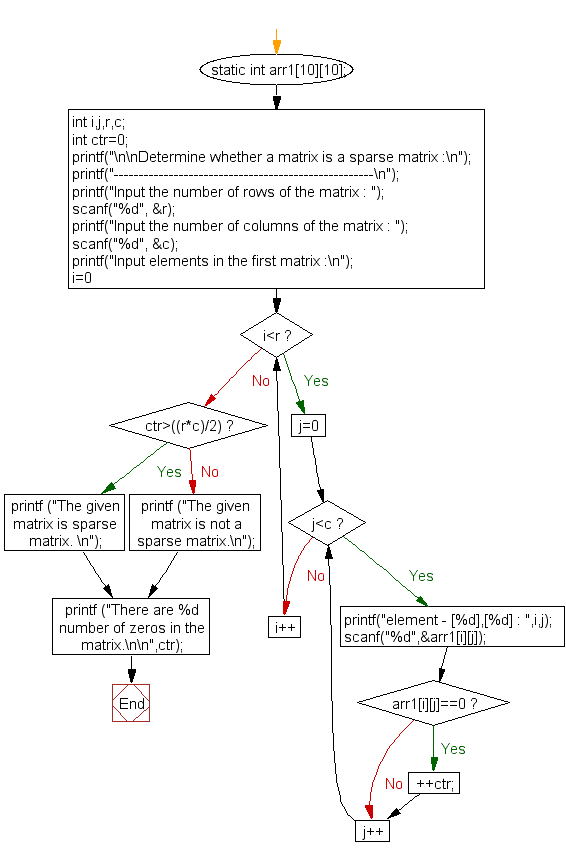
For more Practice: Solve these Related Problems:
- Write a C program to determine if a matrix is sparse by counting zeros and comparing with non-zero elements.
- Write a C program to convert a sparse matrix to its compressed row storage format.
- Write a C program to check if a matrix is sparse and then display the positions of zero elements.
- Write a C program to accept a matrix and determine its sparsity percentage.
C Programming Code Editor:
Previous: Write a program in C to calculate determinant of a 3 x 3 matrix.
Next: Write a program in C to accept two matrices and check whether they are equal.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.