C Exercises: Accept two matrices and check whether they are equal
30. Matrix Equality Check
Write a program in C to accept two matrices and check whether they are equal.
The task is to write a C program that accepts two matrices and checks if they are equal. The program prompts the user to input the dimensions and elements of both matrices, then compares corresponding elements. If all elements are identical, the program will confirm that the matrices are equal.
Visual Presentation:
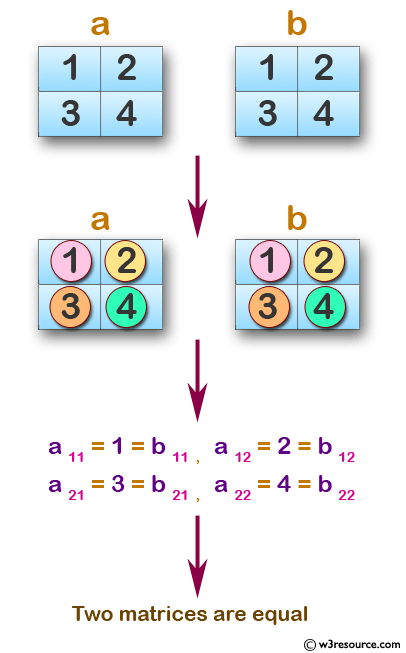
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
int main() {
int arr1[50][50], brr1[50][50];
int i, j, r1, c1, r2, c2, flag = 1;
// Display the purpose of the program
printf("\n\nAccept two matrices and check whether they are equal :\n");
printf("-----------------------------------------------------------\n");
// Input Rows and Columns of the 1st matrix
printf("Input Rows and Columns of the 1st matrix :");
scanf("%d %d", &r1, &c1);
// Input Rows and Columns of the 2nd matrix
printf("Input Rows and Columns of the 2nd matrix :");
scanf("%d %d", &r2, &c2);
// Input elements into the first matrix
printf("Input elements in the first matrix :\n");
for(i = 0; i < r1; i++) {
for(j = 0; j < c1; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &arr1[i][j]);
}
}
// Input elements into the second matrix
printf("Input elements in the second matrix :\n");
for(i = 0; i < r2; i++) {
for(j = 0; j < c2; j++) {
printf("element - [%d],[%d] : ", i, j);
scanf("%d", &brr1[i][j]);
}
}
// Display the first matrix
printf("The first matrix is :\n");
for(i = 0; i < r1; i++) {
for(j = 0; j < c1; j++) {
printf("% 4d", arr1[i][j]);
}
printf("\n");
}
// Display the second matrix
printf("The second matrix is :\n");
for(i = 0; i < r2; i++) {
for(j = 0; j < c2; j++) {
printf("% 4d", brr1[i][j]);
}
printf("\n");
}
// Comparing two matrices for equality
if(r1 == r2 && c1 == c2) {
printf("The Matrices can be compared : \n");
for(i = 0; i < r1; i++) {
for(j = 0; j < c2; j++) {
if(arr1[i][j] != brr1[i][j]) {
flag = 0;
break;
}
}
}
} else {
printf("The Matrices Cannot be compared :\n");
exit(1);
}
// Display whether matrices are equal or not
if(flag == 1)
printf("Two matrices are equal.\n\n");
else
printf("But, two matrices are not equal.\n\n");
return 0;
}
Sample Output:
Accept two matrices and check whether they are equal : ----------------------------------------------------------- Input Rows and Columns of the 1st matrix :2 2 Input Rows and Columns of the 2nd matrix :2 2 Input elements in the first matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [1],[0] : 3 element - [1],[1] : 4 Input elements in the second matrix : element - [0],[0] : 1 element - [0],[1] : 2 element - [1],[0] : 3 element - [1],[1] : 4 The first matrix is : 1 2 3 4 The second matrix is : 1 2 3 4 The Matrices can be compared : Two matrices are equal.
Flowchart:
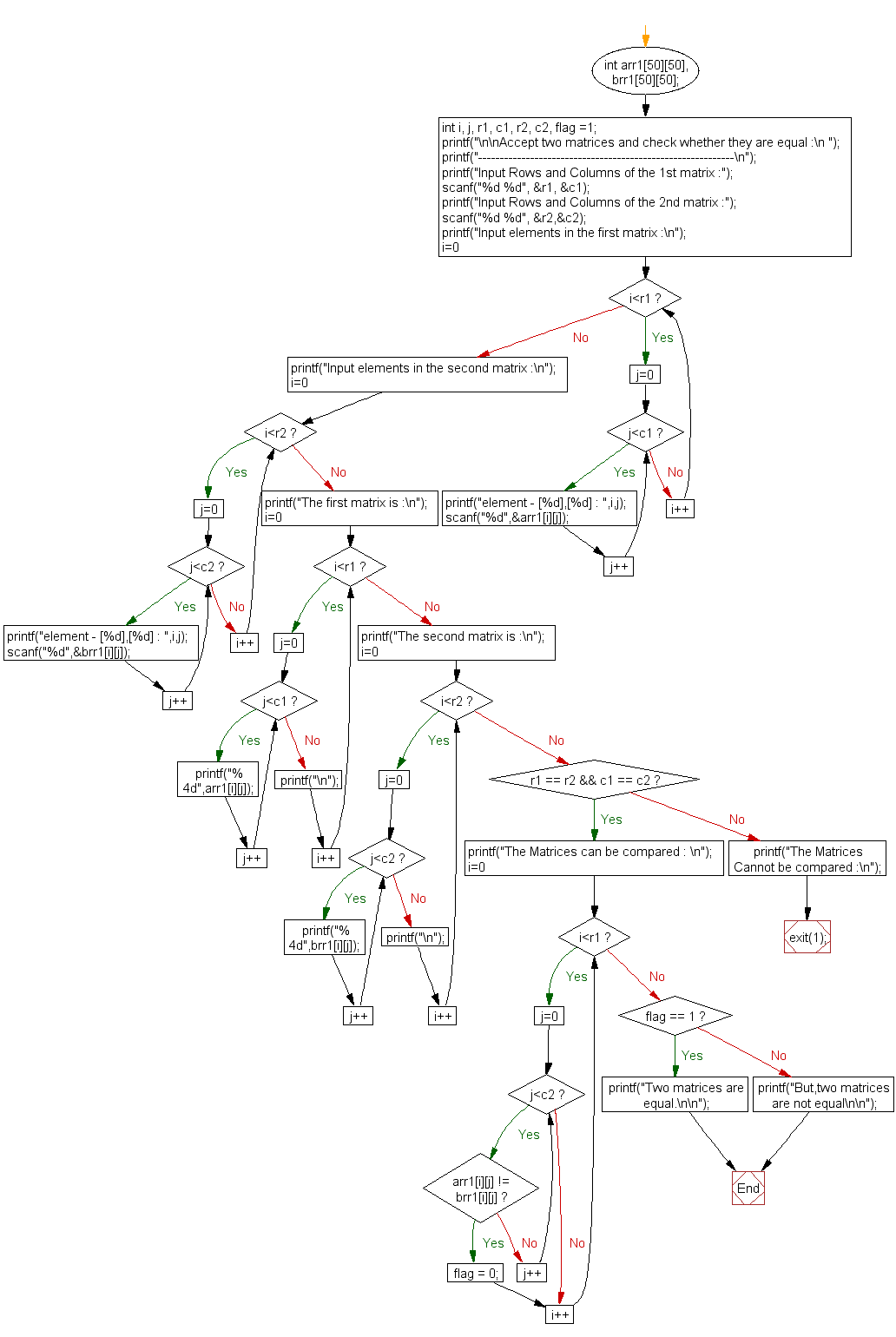
For more Practice: Solve these Related Problems:
- Write a C program to compare two matrices element-wise and output the differing elements.
- Write a C program to check if two matrices are equal using pointer arithmetic.
- Write a C program to accept two matrices and then verify equality using recursion.
- Write a C program to compare two matrices and display a message for each row indicating if they are equal.
C Programming Code Editor:
Previous: Write a program in C to accept a matrix and determine whether it is a sparse matrix.
Next: Write a program in C to check whether a given matrix is an identity matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.