C Exercises: Count the frequency of each element of an array
8. Frequency of Array Elements
Write a program in C to count the frequency of each element of an array.
The task requires writing a C program to count and display the frequency of each element in an array. The program will accept a specified number of integer inputs, store them in an array, and then determine and print how many times each element appears in the array.
Visual Presentation:
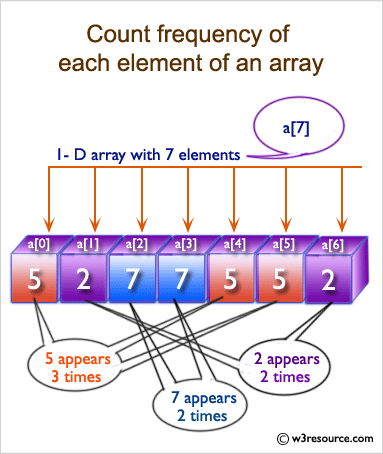
Sample Solution:
C Code:
#include <stdio.h>
int main()
{
int arr1[100], fr1[100];
int n, i, j, ctr;
// Prompt user for input
printf("\n\nCount frequency of each element of an array:\n");
printf("------------------------------------------------\n");
printf("Input the number of elements to be stored in the array :");
scanf("%d", &n);
// Input elements for the array
printf("Input %d elements in the array :\n", n);
for (i = 0; i < n; i++)
{
printf("element - %d : ", i);
scanf("%d", &arr1[i]);
fr1[i] = -1; // Initialize frequency array with -1
}
// Count the frequency of each element in the array
for (i = 0; i < n; i++)
{
ctr = 1; // Initialize counter for each element
for (j = i + 1; j < n; j++)
{
if (arr1[i] == arr1[j])
{
ctr++; // Increment counter for matching elements
fr1[j] = 0; // Mark the duplicate element's frequency as 0
}
}
// If frequency array value is not marked as 0, set it to the counter
if (fr1[i] != 0)
{
fr1[i] = ctr;
}
}
// Print the frequency of each element in the array
printf("\nThe frequency of all elements of the array : \n");
for (i = 0; i < n; i++)
{
if (fr1[i] != 0)
{
printf("%d occurs %d times\n", arr1[i], fr1[i]);
}
}
return 0;
}
Sample Output:
Count frequency of each element of an array: ------------------------------------------------ Input the number of elements to be stored in the array :3 Input 3 elements in the array : element - 0 : 25 element - 1 : 12 element - 2 : 43 The frequency of all elements of array : 25 occurs 1 times 12 occurs 1 times 43 occurs 1 times
Explanation:
printf("Input the number of elements to be stored in the array :"); scanf("%d",&n); printf("Input %d elements in the array :\n",n); for(i=0;i<n;i++) { printf("element - %d : ",i); scanf("%d",&arr1[i]); fr1[i] = -1; } for(i=0; i<n; i++) { ctr = 1; for(j=i+1; j<n; j++) { if(arr1[i]==arr1[j]) { ctr++; fr1[j] = 0; } } if(fr1[i]!=0) { fr1[i] = ctr; } } printf("\nThe frequency of all elements of array : \n"); for(i=0; i<n; i++) { if(fr1[i]!=0) { printf("%d occurs %d times\n", arr1[i], fr1[i]); } }
In the above code -
- The first printf statement prompts the user to input the number of elements they want to store in the array and stores it in the variable n using scanf.
- The second printf statement asks the user to input n number of elements into the array arr1 using a for loop, and stores each input in the corresponding index of the array arr1[i]. It also initializes an array fr1 with -1 for each element.
- The next for loop then iterates over each element in arr1, and calculates its frequency by comparing it to every other element in the array using nested for loops. The variable ctr is used to keep track of the number of occurrences of each element, and the array fr1 is used to store the frequency of each element.
- The final for loop then prints out the frequency of each element in the array using the values stored in arr1 and fr1.
Flowchart:
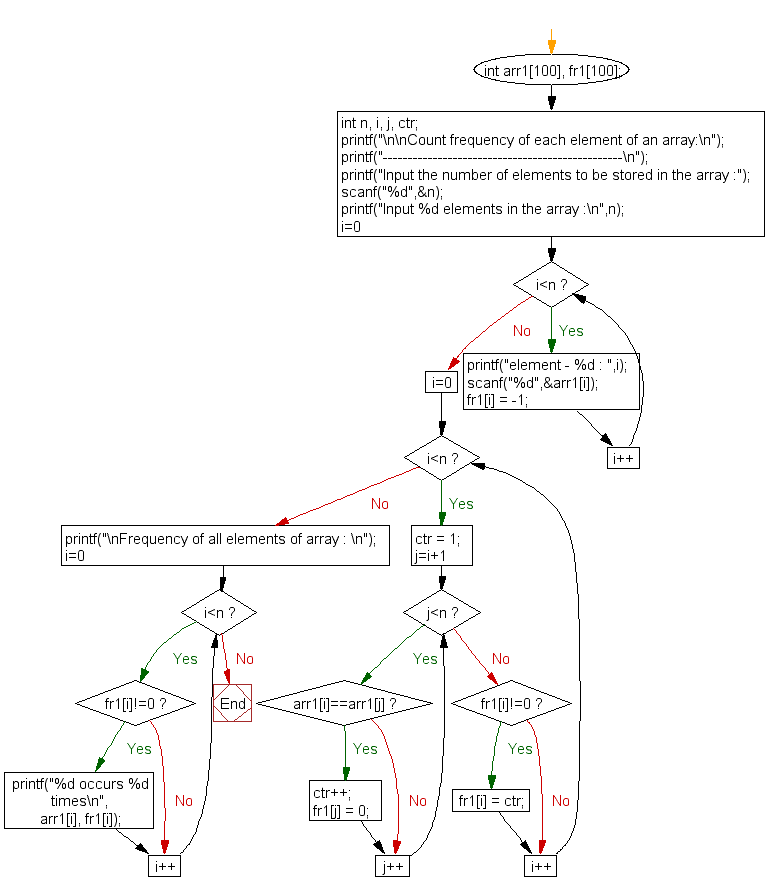
For more Practice: Solve these Related Problems:
- Write a C program to count the frequency of each element in an array using a nested loop.
- Write a C program to compute and print the frequency of elements without using extra space by modifying the original array.
- Write a C program to count frequencies using a hash map implemented with arrays.
- Write a C program to display each unique element and its frequency, then sort the output by frequency.
C Programming Code Editor:
Previous: Write a program in C to merge two arrays of same size sorted in decending order.
Next: Write a program in C to find the maximum and minimum element in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.