C Exercises: Merge two arrays of same size sorted in decending order
C Array: Exercise-7 with Solution
Write a program in C to merge two arrays of the same size sorted in descending order.
This task requires writing a C program to merge two arrays of the same size and sort the resulting array in descending order. The program will take inputs for both arrays, merge them, and then sort and display the merged array in descending order.
Visual Presentation:
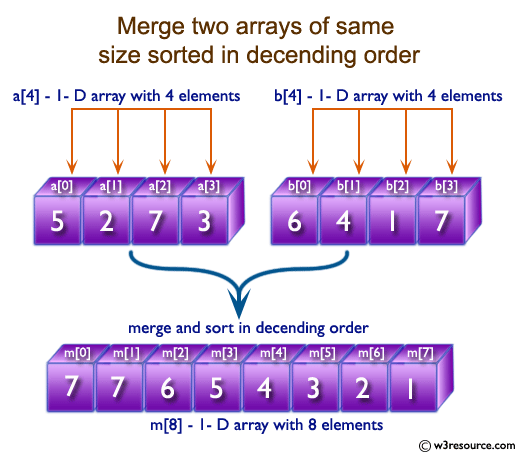
Sample Solution:
C Code:
#include <stdio.h>
int main()
{
int arr1[100], arr2[100], arr3[200];
int s1, s2, s3;
int i, j, k;
printf("\n\nMerge two arrays of same size sorted in decending order.\n");
printf("------------------------------------------------------------\n");
printf("Input the number of elements to be stored in the first array :");
scanf("%d",&s1);
printf("Input %d elements in the array :\n",s1);
for(i=0;i<s1;i++)
{
printf("element - %d : ",i);
scanf("%d",&arr1[i]);
}
printf("Input the number of elements to be stored in the second array :");
scanf("%d",&s2);
printf("Input %d elements in the array :\n",s2);
for(i=0;i<s2;i++)
{
printf("element - %d : ",i);
scanf("%d",&arr2[i]);
}
/* size of merged array is size of first array and size of second array */
s3 = s1 + s2;
/*----------------- insert in the third array------------------------------------*/
for(i=0;i<s1; i++)
{
arr3[i] = arr1[i];
}
for(j=0;j<s2; j++)
{
arr3[i] = arr2[j];
i++;
}
/*----------------- sort the array in decending order ---------------------------*/
for(i=0;i<s3; i++)
{
for(k=0;k<s3-1;k++)
{
if(arr3[k]<=arr3[k+1])
{
j=arr3[k+1];
arr3[k+1]=arr3[k];
arr3[k]=j;
}
}
}
/*--------------- Prints the merged array ------------------------------------*/
printf("\nThe merged array in decending order is :\n");
for(i=0; i<s3; i++)
{
printf("%d ", arr3[i]);
}
printf("\n\n");
return 0;
}
Sample Output:
Merge two arrays of same size sorted in decending order. ------------------------------------------------------------ Input the number of elements to be stored in the first array :3 Input 3 elements in the array : element - 0 : 1 element - 1 : 2 element - 2 : 3 Input the number of elements to be stored in the second array :3 Input 3 elements in the array : element - 0 : 1 element - 1 : 2 element - 2 : 3 The merged array in decending order is : 3 3 2 2 1 1
Explanation:
printf("Input the number of elements to be stored in the first array :"); scanf("%d",&s1); printf("Input %d elements in the array :\n",s1); for(i=0;i<s1;i++) { printf("element - %d : ",i); scanf("%d",&arr1[i]); } printf("Input the number of elements to be stored in the second array :"); scanf("%d",&s2); printf("Input %d elements in the array :\n",s2); for(i=0;i<s2;i++) { printf("element - %d : ",i); scanf("%d",&arr2[i]); } /* size of merged array is size of first array and size of second array */ s3 = s1 + s2; /*----------------- insert in the third array------------------------------------*/ for(i=0;i<s1; i++) { arr3[i] = arr1[i]; } for(j=0;j<s2; j++) { arr3[i] = arr2[j]; i++; } /*----------------- sort the array in decending order ---------------------------*/ for(i=0;i<s3; i++) { for(k=0;k<s3-1;k++) { if(arr3[k]<=arr3[k+1]) { j=arr3[k+1]; arr3[k+1]=arr3[k]; arr3[k]=j; } } } /*--------------- Prints the merged array ------------------------------------*/ printf("\nThe merged array in decending order is :\n"); for(i=0; i<s3; i++) { printf("%d ", arr3[i]); }
In the above code -
- The first printf statement prompts the user to input the number of elements they want to store in the first array and stores it in the variable s1 using scanf.
- The second printf statement asks the user to input s1 number of elements into the first array arr1 using a for loop, and stores each input in the corresponding index of the array arr1[i].
- The third printf statement prompts the user to input the number of elements they want to store in the second array and stores it in the variable s2 using scanf.
- The fourth printf statement asks the user to input s2 number of elements into the second array arr2 using a for loop, and stores each input in the corresponding index of the array arr2[i].
- The next for loop then combines the two arrays into a single array arr3 by copying the elements of arr1 into arr3, and then copying the elements of arr2 into arr3 starting from the index after the last element of arr1.
- The next for loop sorts the elements in arr3 in descending order using a bubble sort algorithm.
- Finally, the last printf statement prints out the merged and sorted arr3.
Flowchart:
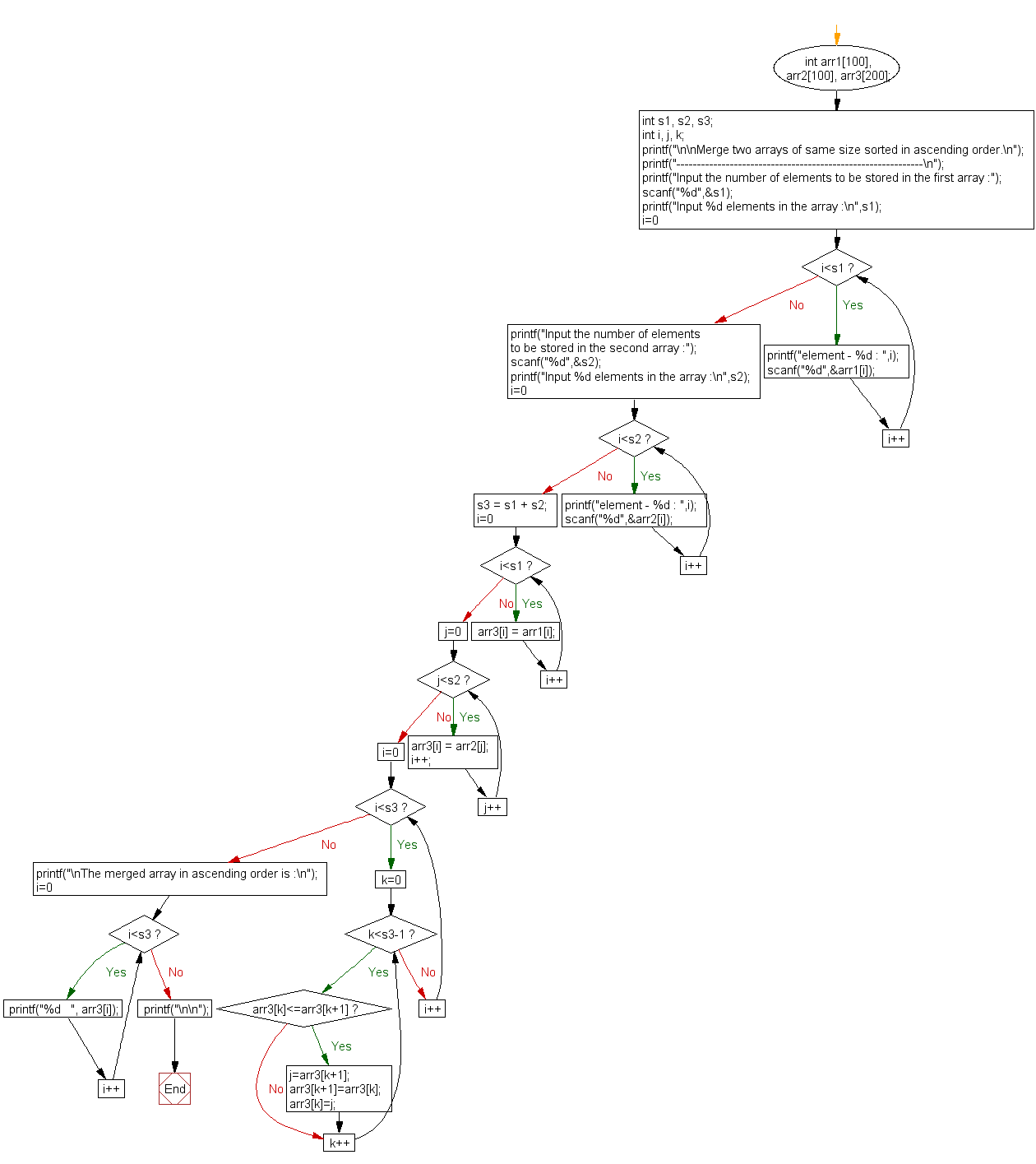
C Programming Code Editor:
Previous: Write a program in C to print all unique elements in an array.
Next: Write a program in C to count the frequency of each element of an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics