C Exercises: Print all unique elements of an array
C Array: Exercise-6 with Solution
Write a program in C to print all unique elements in an array.
This task requires writing a C program to identify and print all unique elements in an array. The program will accept a specified number of integer inputs, store them in an array, and then determine and display the elements that appear only once in the array
Visual Presentation:
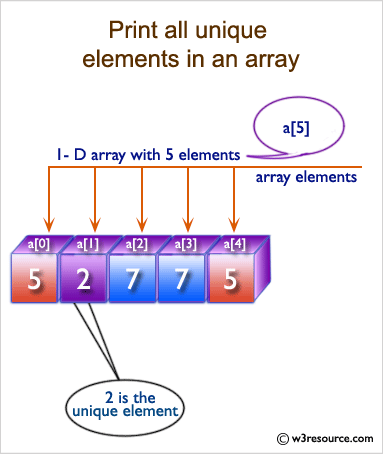
Pseudocode to print all unique elements of an array:
- Take the array size and elements as input from the user.
- Initialize an empty array called distinct.
- For each element in the input array:
- Check if the element is already in the unique array.
- If it is not, add it to the unique array.
- Print the unique array.
Note: We assumes that the input array contains only primitive data types. The algorithm should be modified accordingly if the input array contains objects or complex data types.
Sample Solution-1:
C Code:
#include <stdio.h>
// Main function
int main()
{
int arr1[100], n, ctr = 0; // Declare an array to store integer values, n for array size, and ctr for counting duplicates
int i, j, k; // Declare loop counters
// Prompt the user to input the number of elements to be stored in the array
printf("\n\nPrint all unique elements of an array:\n");
printf("------------------------------------------\n");
printf("Input the number of elements to be stored in the array: ");
scanf("%d", &n);
// Prompt the user to input n elements into the array
printf("Input %d elements in the array :\n", n);
for (i = 0; i < n; i++)
{
printf("element - %d : ", i);
scanf("%d", &arr1[i]); // Read the input and store it in the array
}
// Print unique elements in the array
printf("\nThe unique elements found in the array are: \n");
for (i = 0; i < n; i++)
{
ctr = 0; // Reset the counter for each element
for (j = 0, k = n; j < k + 1; j++)
{
/* Increment the counter when the search value is duplicate. */
if (i != j)
{
if (arr1[i] == arr1[j])
{
ctr++;
}
}
}
if (ctr == 0)
{
printf("%d ", arr1[i]); // Print the unique element
}
}
printf("\n\n");
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Print all unique elements of an array: ------------------------------------------ Input the number of elements to be stored in the array: 4 Input 4 elements in the array : element - 0 : 3 element - 1 : 2 element - 2 : 2 element - 3 : 5 The unique elements found in the array are: 3 5
Explanation:
printf("Input the number of elements to be stored in the array: "); scanf("%d",&n); printf("Input %d elements in the array :\n",n); for(i=0;i<n;i++) { printf("element - %d : ",i); scanf("%d",&arr1[i]); } printf("\nThe unique elements found in the array are: \n"); for(i=0; i<n; i++) { ctr=0; for(j=0,k=n; j<k+1; j++) { /*Increment the counter when the seaarch value is duplicate.*/ if (i!=j) { if(arr1[i]==arr1[j]) { ctr++; } } } if(ctr==0) { printf("%d ",arr1[i]); } }
In the above code -
- The first printf statement prompts the user to input the number of elements they want to store in the array and stores it in the variable n using scanf.
- The second printf statement asks the user to input n number of elements into the array arr1 using a for loop, and stores each input in the corresponding index of the array arr1[i].
- The third printf statement simply prints out a message indicating that the unique elements found in the array will be displayed.
- The next for loop iterates over each element in arr1 and checks for uniqueness by comparing it to every other element in the array using nested for loops. The variable ctr is used to keep track of the number of duplicates found.
- If no duplicates are found, the element is considered unique and is printed out using printf() function.
Flowchart:
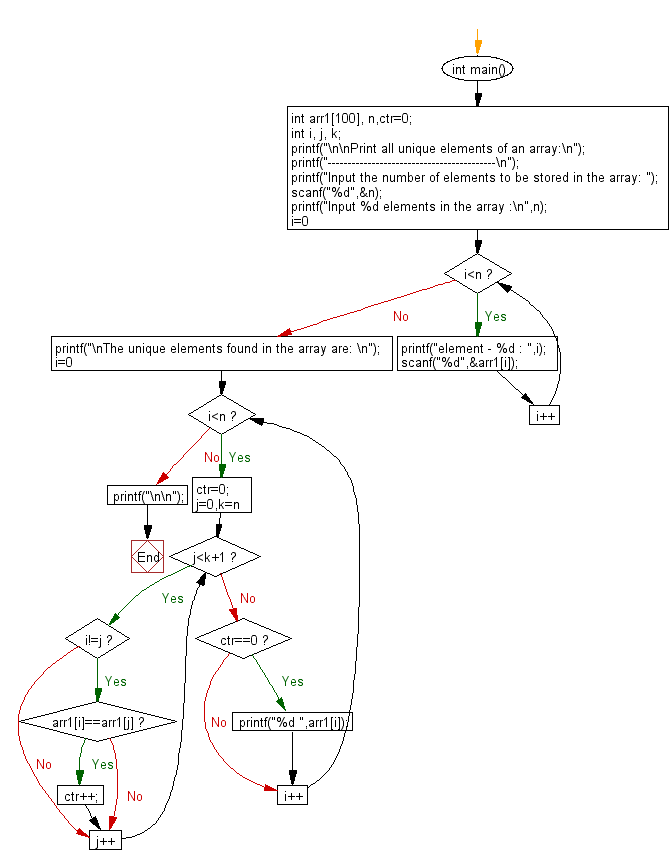
Sample Solution-2:
C# Sharp Code:
#include <stdio.h>
#include
// Main function
int main() {
int n, i, j;
// Prompt the user to input the size of the array
printf("Input the size of the array: ");
scanf("%d", &n);
int nums[n]; // Declare an array to store integer values
bool is_Unique[n]; // Declare an array to store whether each element is unique or not
// Loop to read input values into the array and initialize is_Unique array
for (i = 0; i < n; i++) {
scanf("%d", &nums[i]);
is_Unique[i] = true; // Assume all elements are unique initially
}
// Loop to check for duplicate elements
for (i = 0; i < n; i++) {
for (j = i + 1; j < n; j++) {
if (nums[i] == nums[j]) {
is_Unique[i] = false; // Mark the current and duplicate element as not unique
is_Unique[j] = false;
}
}
}
// Print unique elements in the array
printf("Unique elements in the array are: ");
for (i = 0; i < n; i++) {
if (is_Unique[i]) {
printf("%d ", nums[i]); // Print the unique element
}
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Input the size of the array: 5 1 1 1 2 3 Unique elements in the array are: 2 3
C Programming Code Editor:
Previous: Write a program in C to count a total number of duplicate elements in an array.
Next: Write a program in C to merge two arrays of same size sorted in decending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics