C Exercises: Find the maximum and minimum element in an array
9. Find Maximum & Minimum
Write a program in C to find the maximum and minimum elements in an array.
The task involves writing a C program to find and display the maximum and minimum elements in an array. The program will take a specified number of integer inputs, store them in an array, and then determine and print the highest and lowest values among the elements.
Visual Presentation:
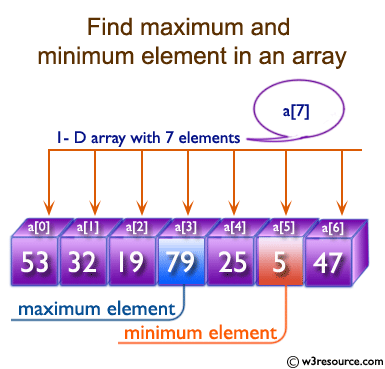
Sample Solution:
C Code:
#include <stdio.h>
int main()
{
int arr1[100];
int i, mx, mn, n;
// Prompt user for input
printf("\n\nFind maximum and minimum element in an array :\n");
printf("--------------------------------------------------\n");
printf("Input the number of elements to be stored in the array :");
scanf("%d", &n);
// Input elements for the array
printf("Input %d elements in the array :\n", n);
for (i = 0; i < n; i++)
{
printf("element - %d : ", i);
scanf("%d", &arr1[i]);
}
// Initialize max (mx) and min (mn) with the first element of the array
mx = arr1[0];
mn = arr1[0];
// Traverse the array to find the maximum and minimum elements
for (i = 1; i < n; i++)
{
// Update mx if the current element is greater
if (arr1[i] > mx)
{
mx = arr1[i];
}
// Update mn if the current element is smaller
if (arr1[i] < mn)
{
mn = arr1[i];
}
}
// Print the maximum and minimum elements
printf("Maximum element is : %d\n", mx);
printf("Minimum element is : %d\n\n", mn);
return 0;
}
Sample Output:
Find maximum and minimum element in an array : -------------------------------------------------- Input the number of elements to be stored in the array :3 Input 3 elements in the array : element - 0 : 45 element - 1 : 25 element - 2 : 21 Maximum element is : 45 Minimum element is : 21
Explanation:
printf("Input the number of elements to be stored in the array :"); scanf("%d",&n); printf("Input %d elements in the array :\n",n); for(i=0;i<n;i++) { printf("element - %d : ",i); scanf("%d",&arr1[i]); } mx = arr1[0]; mn = arr1[0]; for(i=1; i<n; i++) { if(arr1[i]>mx) { mx = arr1[i]; } if(arr1[i]<mn) { mn = arr1[i]; } } printf("Maximum element is : %d\n", mx); printf("Minimum element is : %d\n\n", mn);
In the above code -
- The first printf statement prompts the user to input the number of elements they want to store in the array and stores it in the variable n using scanf.
- The second printf statement asks the user to input n number of elements into the array arr1 using a for loop, and stores each input in the corresponding index of the array arr1[i].
- The next for loop then iterates over each element in arr1 and finds the maximum and minimum elements in the array by comparing it to every other element in the array using if statements. The variables mx and mn are used to store the maximum and minimum elements, respectively.
- Finally, the last printf statement prints out the maximum and minimum elements found.
Flowchart:
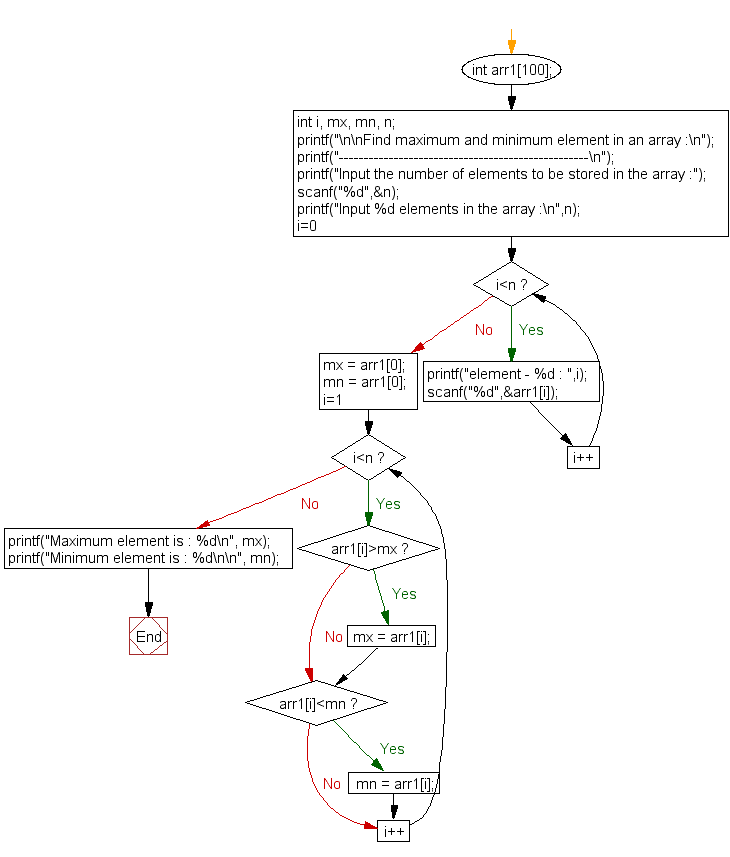
For more Practice: Solve these Related Problems:
- Write a C program to find the maximum and minimum elements in an array using a single loop and pointer arithmetic.
- Write a C program to determine the max and min values in an array recursively.
- Write a C program to find the maximum and minimum elements and then swap them.
- Write a C program to find max and min values in an array without using any conditional statements.
C Programming Code Editor:
Previous: Write a program in C to count the frequency of each element of an array.
Next: Write a program in C to separate odd and even integers in separate arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.