C Exercises: Accept two integers and return true if either one is 5 or their sum or difference is 5
C-programming basic algorithm: Exercise-18 with Solution
Write a C program that accepts two integers and checks whether either one of them is 5 or their sum or difference is 5. If any of these conditions are met, the program returns true. Otherwise, it returns false.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with two integer parameters
int test(int x, int y);
int main(void){
// Print the result of calling 'test' with different integer values and format the output
printf("%d",test(5, 4));
printf("\n%d",test(4, 3));
printf("\n%d",test(1, 4));
}
// Function definition for 'test'
int test(int x, int y)
{
// Check if either 'x' is 5, 'y' is 5, the sum of 'x' and 'y' is 5, or the absolute difference between 'x' and 'y' is 5
if (x == 5 || y == 5 || x + y == 5 || abs(x - y) == 5) {
return 1; // If any of the conditions are met, return 1 (true)
} else {
return 0; // If none of the conditions are met, return 0 (false)
}
}
Sample Output:
1 0 1
Explanation:
int test(int x, int y) { return x == 5 || y == 5 || x + y == 5 || abs(x - y) == 5; }
The function takes two integer arguments x and y. It returns a boolean value based on whether either x or y is equal to 5, the sum of x and y is equal to 5, or the absolute difference between x and y is equal to 5.
- If x or y is equal to 5, the function returns true (1).
- If the sum of x and y is equal to 5, the function returns true.
- If the absolute difference between x and y is equal to 5, the function returns true.
- If none of the above conditions are met, the function returns false (0).
Time complexity and space complexity:
The time complexity of the function is O(1), as the operations performed in the function take a constant amount of time.
The space complexity of the function is O(1), as it only declares two integer variables and does not dynamically allocate any memory.
Pictorial Presentation:
Flowchart:
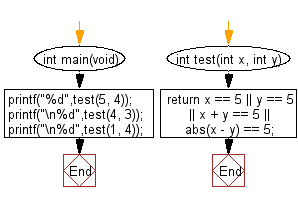
C Programming Code Editor:
Previous: Write a C program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
Next: Write a C program to test if a given non-negative number is a multiple of 13 or it is one more than a multiple of 13.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics