C Exercises: Test whether a non-negative number is a multiple of 13 or it is one more than a multiple of 13
19. Multiple of 13 or One More
Write a C program that checks if a given non-negative integer is a multiple of 13 or one more than a multiple of 13. For example, if the given integer is 26 or 27, the program will return true, but if it is 25 or 28, the program will return false.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with an integer parameter 'n'
int test(int n);
int main(void){
// Print the result of calling 'test' with different integer values and format the output
printf("%d",test(13));
printf("\n%d",test(14));
printf("\n%d",test(27));
printf("\n%d",test(41));
}
// Function definition for 'test'
int test(int n)
{
// Check if 'n' is divisible by 13 or if the remainder of 'n' divided by 13 is 1
if (n % 13 == 0 || n % 13 == 1) {
return 1; // If any of the conditions are met, return 1 (true)
} else {
return 0; // If none of the conditions are met, return 0 (false)
}
}
Sample Output:
1 1 1 0
Explanation:
int test(int n) { return n % 13 == 0 || n % 13 == 1; }
The function takes an integer n as input and returns a boolean value. It checks if n is a multiple of 13 or if n is one more than a multiple of 13. If either condition is true, it returns true; otherwise, it returns false.
- Write a C program to check if a non-negative integer is a multiple of 17 or one less than a multiple of 17.
- Write a C program to verify if a given number is a multiple of 12 or two more than a multiple of 12.
- Write a C program that checks if a number is either a multiple of 13 or 2 more than a multiple of 13.
- Write a C program to check if an integer is one less than a multiple of 14 or exactly a multiple of 14.
Time complexity and space complexity:
The time complexity of the function is O(1) because it performs a simple arithmetic operation (modulus) on a single input integer.
The space complexity of the function is O(1) because it only uses a few integer variables to store the input and intermediate calculations.
Flowchart:
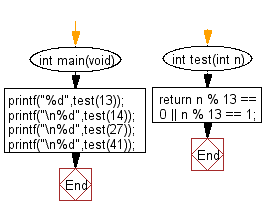
For more Practice: Solve these Related Problems:
C Programming Code Editor:
Previous: Write a C program that accept two integers and return true if either one is 5 or their sum or difference is 5.
Next: Write a C program to check whether a given non-negative number is a multiple of 3 or 7, but not both.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.