C Exercises: Compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30
17. Sum Range 10 to 20 to 30
Write a C program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with two integer parameters
int test(int a, int b);
int main(void){
// Print the result of calling 'test' with different integer values and format the output
printf("%d",test(12, 17));
printf("\n%d",test(2, 17));
printf("\n%d",test(22, 17));
printf("\n%d",test(20, 0));
}
// Function definition for 'test'
int test(int a, int b)
{
// Check if the sum of 'a' and 'b' is between 10 and 20 (inclusive)
if (a + b >= 10 && a + b <= 20) {
return 30; // If the condition is met, return 30
} else {
return a + b; // If not, return the sum of 'a' and 'b'
}
}
Sample Output:
29 30 39 30
Explanation:
int test(int a, int b) { return a + b >= 10 && a + b <= 20 ? 30 : a + b; }
The said function takes two integers 'a' and 'b' as input parameters and returns an integer value. The function first checks if the sum of 'a' and 'b' is between 10 and 20 (inclusive). If yes, then it returns the value 30, otherwise, it returns the sum of 'a' and 'b'.
Time complexity and space complexity:
The time complexity of the function is O(1) as the function has constant time complexity, as the execution time is not dependent on the size of the inputs.
The space complexity of the function is O(1) as the function has constant space complexity and it does not use any extra space apart from the input parameters and the return value.
Pictorial Presentation:
Flowchart:
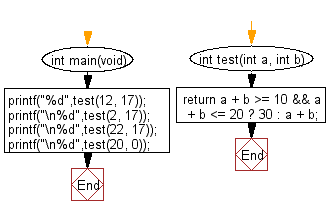
For more Practice: Solve these Related Problems:
- Write a C program to compute the sum of two numbers, and if the sum is between 15 and 25, return 50.
- Write a C program that computes the sum of two integers and returns a fixed value if the sum is prime.
- Write a C program to add two numbers and, if the sum is a multiple of 5, return the product instead.
- Write a C program to compute the sum of two integers and return 0 if the sum falls within a user-defined range.
C Programming Code Editor:
Previous: Write a C program to check if a triple is presents in an array of integers or not. If a value appears three times in a row in an array it is called a triple.
Next: Write a C program that accept two integers and return true if either one is 5 or their sum or difference is 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.