C Exercises: Check if a triple is presents in an array of integers or not
16. Triple Occurrence Check
Write a C program to check if a triple is present in an array of integers or not. If a value appears three times in a row in an array it is called a triple.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with an array of integers and its size as parameters
int test(int nums[], int arr_size);
int main(void){
int arr_size; // Declare an integer variable to store the size of the array
// Initialize an integer array 'array1' with given values
int array1[] = {1, 1, 2, 2, 1};
arr_size = sizeof(array1)/sizeof(array1[0]); // Calculate the size of 'array1'
printf("%d",test(array1, arr_size)); // Call the 'test' function with 'array1' and its size, and print the result
// Initialize another integer array 'array2' with given values
int array2[] = {1, 1, 2, 1, 2, 3};
arr_size = sizeof(array2)/sizeof(array2[0]); // Calculate the size of 'array2'
printf("\n%d",test(array2, arr_size)); // Call the 'test' function with 'array2' and its size, and print the result
// Initialize yet another integer array 'array3' with given values
int array3[] = {1, 1, 1, 2, 2, 2, 1 };
arr_size = sizeof(array3)/sizeof(array3[0]); // Calculate the size of 'array3'
printf("\n%d",test(array3, arr_size)); // Call the 'test' function with 'array3' and its size, and print the result
}
// Function definition for 'test'
int test(int nums[], int arr_size)
{
// Iterate through the elements of the array 'nums'
for (int i = 0; i < arr_size - 1; i++)
{
// Check if the current element is equal to the next two consecutive elements
if(nums[i] == nums[i + 1] && nums[i + 2] == nums[i])
return 1; // If the condition is met, return 1 (true)
}
return 0; // If no match is found, return 0 (false)
}
Sample Output:
0 0 1
Explanation:
int test(int nums[], int arr_size) { for (int i = 0; i < arr_size - 1; i++) { if (nums[i] == nums[i + 1] && nums[i + 2] == nums[i]) return 1; } return 0; }
The above function takes an array of integers and its size as input. It checks if the array contains any sequence of three integers where the first and third integers are the same. If such a sequence is found, the function returns 1, otherwise, it returns 0.
Time complexity and space complexity:
The time complexity of the given function is O(n), where n is the size of the input array. This is because the function iterates over the array once to check for the required sequence of integers.
The space complexity of the given function is O(1), as it uses a constant amount of additional space to store the loop variable and the result variable.
Pictorial Presentation:
Flowchart:
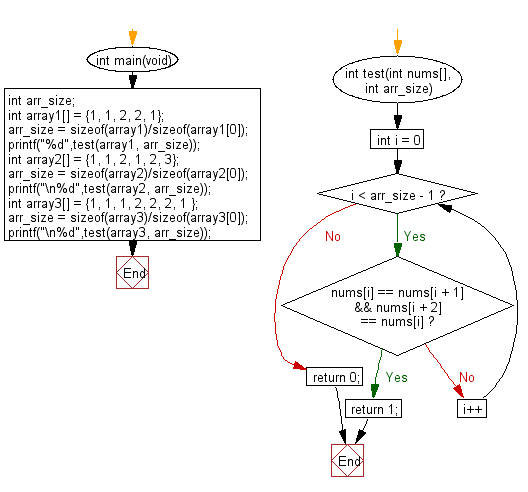
For more Practice: Solve these Related Problems:
- Write a C program to detect if an array contains a double (two consecutive identical elements).
- Write a C program to count how many times a sequence of three consecutive even numbers occurs in an array.
- Write a C program to check if an array contains any number repeated exactly three times, not necessarily consecutively.
- Write a C program to verify if any element in an array appears three times with exactly one different number between them.
C Programming Code Editor:
Previous: Write a C program to count the number of two 5's are next to each other in an array of integers. Also count the situation where the second 5 is actually a 6.
Next: Write a C program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.