C Exercises: Count the number of two 5's are next to each other in an array of integers
15. Count Adjacent 5's with 6 Twist
Write a C program to count the number of 5's adjacent to each other in an array of integers. Consider the situation where the second 5 is actually a 6.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with an array of integers and its size as parameters
int test(int nums[], int arr_size);
int main(void){
int arr_size; // Declare an integer variable to store the size of the array
// Initialize an integer array 'array1' with given values
int array1[] = {5, 5, 2 };
arr_size = sizeof(array1)/sizeof(array1[0]); // Calculate the size of 'array1'
printf("%d",test(array1, arr_size)); // Call the 'test' function with 'array1' and its size, and print the result
// Initialize another integer array 'array2' with given values
int array2[] = {5, 5, 2, 5, 5 };
arr_size = sizeof(array2)/sizeof(array2[0]); // Calculate the size of 'array2'
printf("\n%d",test(array2, arr_size)); // Call the 'test' function with 'array2' and its size, and print the result
// Initialize yet another integer array 'array3' with given values
int array3[] = {5, 6, 2, 9 };
arr_size = sizeof(array3)/sizeof(array3[0]); // Calculate the size of 'array3'
printf("\n%d",test(array3, arr_size)); // Call the 'test' function with 'array3' and its size, and print the result
}
// Function definition for 'test'
int test(int nums[], int arr_size)
{
int ctr = 0; // Initialize a counter variable to keep track of occurrences
// Iterate through the elements of the array 'nums'
for (int i = 0; i < arr_size - 1; i++)
{
// Check if the current element is 5 and the next element is either 5 or 6
if (nums[i] == 5 && (nums[i + 1] == 5 || nums[i + 1] == 6))
ctr++; // If the condition is met, increment the counter
}
return ctr; // Return the final count
}
Sample Output:
1 2 1
Explanation:
int test(int nums[], int arr_size) { int ctr = 0; for (int i = 0; i < arr_size - 1; i++) { if (nums[i] == 5 && (nums[i + 1] == 5 || nums[i + 1] == 6)) ctr++; } return ctr; }
This function takes an array of integers and its size as input, and counts the number of times the pattern 5, 5 or 5, 6 appears in the array.
It initializes a counter variable ctr to 0, and then loops through the array from index 0 to index arr_size - 2 (since it is checking the current element and the next element). If the current element is 5 and the next element is either 5 or 6, the counter is incremented. After the loop, the function returns the counter value.
Time complexity and space complexity:
The time complexity of this function is O(n), where n is the size of the input array. This is because the function iterates through the array once, checking each element and incrementing the counter if the pattern is found.
The space complexity of this function is O(1), because it only uses a constant amount of extra space to store the counter variable.
Pictorial Presentation:
Flowchart:
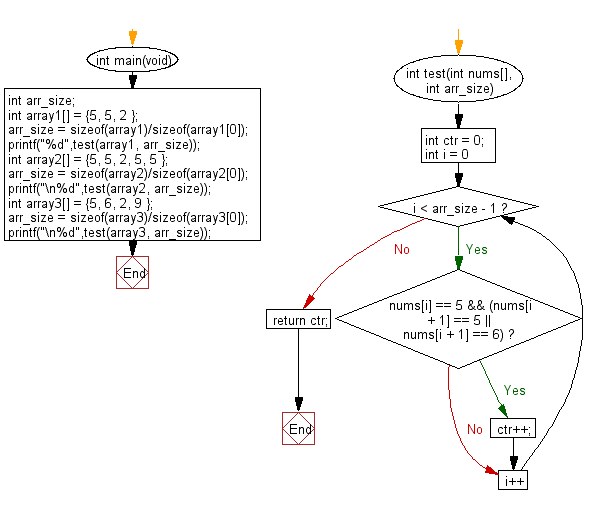
For more Practice: Solve these Related Problems:
- Write a C program to count occurrences of adjacent numbers where the second is one greater than the first.
- Write a C program to count pairs of consecutive numbers that add up to 10 in an array.
- Write a C program to detect and count pairs of identical adjacent elements, ignoring a subsequent modifier value.
- Write a C program to count adjacent pairs in an array where the first number is 3 and the second is either 3 or 4.
C Programming Code Editor:
Previous: Write a C program to check whether the sequence of numbers 1, 2, 3 appears in a given array of integers somewhere.
Next: Write a C program to check if a triple is presents in an array of integers or not. If a value appears three times in a row in an array it is called a triple.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.