C Exercises: Check two given integers and return the value whichever value is nearest to 13 without going over
32. Nearest to 13 Without Crossing
Write a C program to check two given integers and return the one nearest to 13 without crossing over. Return 0 if both numbers go over.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int x, int y);
int main(void) {
// Printing the results of calling 'test' function with different arguments
printf("%d", test(4, 5));
printf("\n%d", test(7, 12));
printf("\n%d", test(10, 13));
printf("\n%d", test(17, 33));
}
// Definition of the 'test' function
int test(int x, int y) {
// Checking conditions and returning appropriate values based on comparisons
if (x > 13 && y > 13) return 0;
if (x <= 13 && y > 13) return x;
if (y <= 13 && x > 13) return y;
// If none of the above conditions are met, return the greater of 'x' and 'y'
return x > y ? x : y;
}
Sample Output:
5 12 13 0
Explanation:
int test(int x, int y) { if (x > 13 && y > 13) return 0; if (x <= 13 && y > 13) return x; if (y <= 13 && x > 13) return y; return x > y ? x : y; }
The above function takes two integers x and y as input parameters and performs the following operations:
- If both x and y are greater than 13, the function returns 0.
- If x is less than or equal to 13 and y is greater than 13, the function returns x.
- If y is less than or equal to 13 and x is greater than 13, the function returns y.
- If both x and y are less than or equal to 13, the function returns the larger of the two values.
- Write a C program to find the integer closest to 20 that does not exceed it; return 0 if both are above 20.
- Write a C program that checks two integers and returns the one closest to 10 without going over, otherwise return -1.
- Write a C program to compare two numbers and return the one nearest to 15 without exceeding it, with a tie returning 0.
- Write a C program to determine which of two integers is closest to 13, only if neither is greater than 13.
Time complexity and space complexity:
Time complexity: O(1), as the function has a constant number of operations, regardless of the input size.
Space complexity: O(1), as the function uses a constant amount of memory, regardless of the input size.
Pictorial Presentation:
Flowchart:
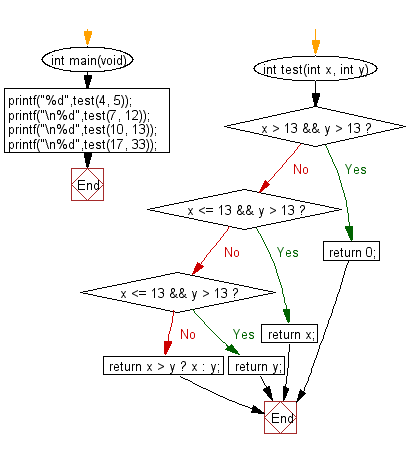
For more Practice: Solve these Related Problems:
C Programming Code Editor:
Previous: Write a C program to compute the sum of the three given integers. However, if any of the values is in the range 10..20 inclusive then that value counts as 0, except 13 and 17.
Next: Write a C program to check three given integers (small, medium and large) and return true if the difference between small and medium and the difference between medium and large is same.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.